Edge Computing with WebAssembly
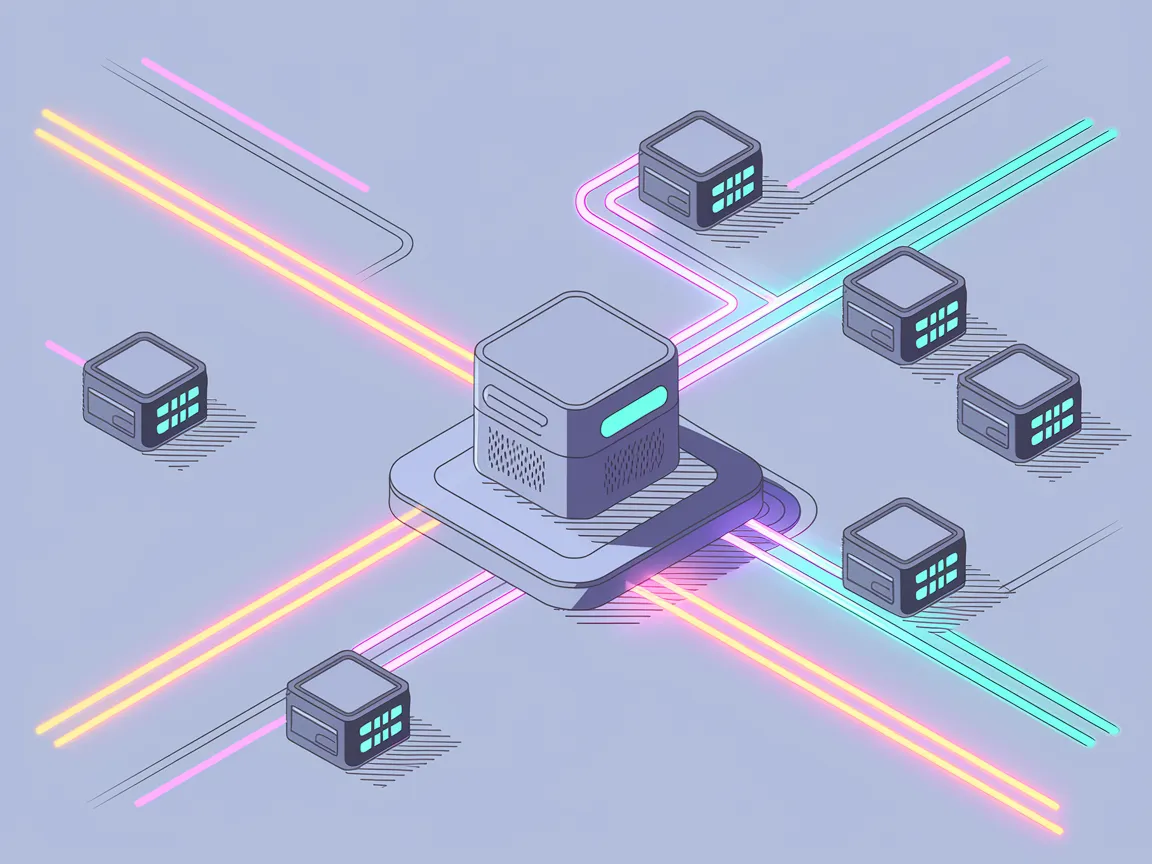
Article
Abstract
WebAssembly (Wasm) has gained traction as a revolutionary technology enabling efficient computation across platforms. In the context of edge computing, it allows for fast, secure execution of applications with minimal overhead. This tutorial provides a comprehensive guide on implementing WebAssembly in edge environments, aimed at both beginners and advanced practitioners. It covers the essential prerequisites, detailed explanations of core concepts, and an implementation guide complete with practical examples.
Key Takeaways:
Efficiency: WebAssembly offers near-native performance, making it a suitable choice for edge computing applications.
Language Flexibility: With support for multiple programming languages, developers can leverage existing codebases.
Deployment: Simple deployment processes for various runtime environments facilitate rapid technology adoption.
Security: WebAssembly provides a sandboxed execution environment, increasing the security of edge applications.
Real-world Applications: As demonstrated by industry case studies, WebAssembly is already enhancing operational efficiency for companies like Amazon and Adobe.
Prerequisites
Tools & Versions
WasmEdge: Version 0.14.1 or later
Rust: Installed via rustup
Docker: For containerization of applications
Setup Instructions
Install Rust:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Install WasmEdge:
curl https://get.wasmer.io -sSfL | sh
Introduction to WebAssembly
WebAssembly is a binary instruction format designed for safe and efficient execution. It allows developers to run compiled code on the web and other environments while ensuring performance and security. For instance, edge computing can benefit significantly from the small binaries produced by compiling code to WebAssembly, enabling quick deployments and execution on resource-constrained devices like IoT sensors.
In real-world applications, companies like Adobe have migrated parts of their rendering engines to WebAssembly, achieving better performance while reducing server load.
Implementation Guide
Step 1: Setting Up Your Environment
Install the necessary tools as mentioned in the prerequisites.
Verify the installations:
wasmedge --version rustc --version
Step 2: Create a Basic Wasm Application
Create a new Rust project:
cargo new edge-app --lib cd edge-app
Add the WebAssembly target:
rustup target add wasm32-wasi
Update
Cargo.toml
:[lib] crate-type = ["cdylib"]
Step 3: Write the Application Code
Create a file named src/lib.rs
and include the following code which processes data:
#[no_mangle]
pub extern "C" fn process_data(input: *const u8, length: usize) -> *const u8 {
let slice = unsafe { std::slice::from_raw_parts(input, length) };
let output: Vec<u8> = slice.iter().map(|&b| b.to_ascii_uppercase()).collect();
output.into_boxed_slice().into_raw()
}
Step 4: Compile and Run
To compile the project:
cargo build --target wasm32-wasi --release
Run the application in WasmEdge:
wasmedge target/wasm32-wasi/release/edge_app.wasm
Code Samples
Sample 1: Image Processing in Edge Applications
#[no_mangle]
pub extern "C" fn resize_image(data: *const u8, width: usize, height: usize, new_width: usize, new_height: usize) -> *const u8 {
// Logic to resize the image
let original = unsafe { std::slice::from_raw_parts(data, width * height * 4) };
// Resize logic goes here
}
Sample 2: Error Handling
#[no_mangle]
pub extern "C" fn safe_divide(numerator: f64, denominator: f64) -> f64 {
if denominator == 0.0 {
eprintln!("Error: Division by zero");
return f64::NAN; // Return NaN in error case
}
numerator / denominator
}
Sample 3: File Handling
#[no_mangle]
pub extern "C" fn read_file(file_path: *const u8) -> *const u8 {
let path = unsafe { std::ffi::CStr::from_ptr(file_path).to_string_lossy().into_owned() };
// Complete logic to read the file and return data
}
Common Challenges
Compilation Errors: Ensure all dependencies in
Cargo.toml
are correctly specified to avoid compilation failures.Performance Bottlenecks: Profile your application and optimize logic, especially for CPU-intensive tasks.
Integration Issues with Existing Systems: Start by creating small, isolated Wasm modules before expanding their functionality.
Advanced Techniques
Optimization Strategy 1: Minifying Wasm Code
Utilize tools like wasm-opt
to decrease the size of the WebAssembly output for faster load times.
Optimization Strategy 2: Using the WebAssembly System Interface (WASI)
Leverage WASI to interact with the operating environment, allowing WebAssembly modules to perform actions like file I/O securely.
Benchmarking
This section should include specific benchmarks comparing WasmEdge and other runtimes (e.g., Node.js) on metrics like cold start time and memory usage.
Industry Applications
Adobe Rendering Engines: Transitioning to WebAssembly for processing complex graphical tasks.
Amazon Transcoding Services: Utilizing Wasm for efficient media processing at the edge.
IoT Device Management: Edge computing applications in agriculture using WebAssembly for data processing.
Conclusion
WebAssembly for edge computing showcases significant potential for enhancing application performance, scalability, and security. As companies adopt this technology, ongoing innovations will shape its future trajectory, potentially leading to broader use cases across various industries.
References
WebAssembly as a Common Layer for the Cloud-edge Continuum. Link
FunLess: Functions-as-a-Service for Private Edge Cloud Systems. Link
Cyber-physical WebAssembly: Secure Hardware Interfaces. Link
Research on WebAssembly Runtimes: A Survey. Link
GoldFish: Serverless Actors with Short-Term Memory State. Link
Article Info
Engage
Table of Contents
- Abstract
- Prerequisites
- Introduction to WebAssembly
- Implementation Guide
- Step 1: Setting Up Your Environment
- Step 2: Create a Basic Wasm Application
- Step 3: Write the Application Code
- Step 4: Compile and Run
- Code Samples
- Common Challenges
- Advanced Techniques
- Optimization Strategy 1: Minifying Wasm Code
- Optimization Strategy 2: Using the WebAssembly System Interface (WASI)
- Benchmarking
- Industry Applications
- Conclusion
- References