ESP32-CAM Implementation Guide: Building Low-Cost Edge Vision Systems
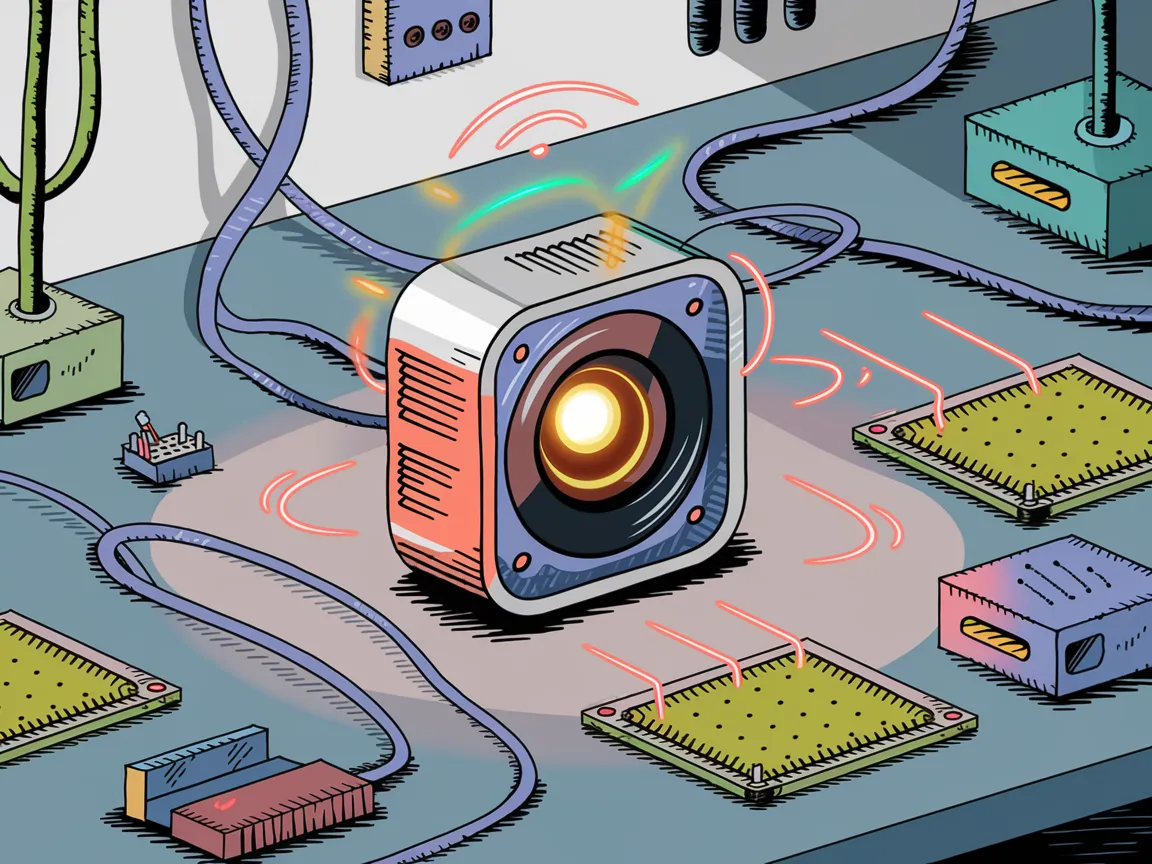
Article
The ESP32-CAM has revolutionized the accessibility of computer vision applications by providing a remarkably affordable solution for edge-based image processing. At approximately $10, this small yet powerful module combines an ESP32 microcontroller with a camera and microSD card slot, making it an ideal platform for IoT applications requiring visual capabilities. This guide covers everything you need to know to implement ESP32-CAM in your projects - from initial setup to advanced applications like motion detection, face recognition, and cloud integration.
Hardware Overview
ESP32-CAM Specifications
Processor: ESP32-S chip (dual-core 32-bit CPU, 240MHz)
Wi-Fi: 2.4GHz 802.11 b/g/n
Bluetooth: Bluetooth 4.2
Memory: 520KB SRAM + 4MB PSRAM
Storage: Supports microSD card up to 32GB
Camera: OV2640 2-megapixel sensor (up to 1600×1200 resolution)
GPIO Pins: 10 accessible GPIO pins
Power Supply: 5V (typical consumption: 180mA)
Size: 40mm x 27mm x 4.5mm
Required Components
ESP32-CAM module
FTDI programmer or USB-to-TTL converter (for programming)
MicroSD card (optional, for storing images)
5V power supply
Jumper wires
Breadboard (for prototyping)
External antenna (optional, for improved range)
GPIO Pin Functions
Pin | Function | Notes |
---|---|---|
GPIO 0 | Boot mode selection | Must be pulled low during programming |
GPIO 1 | TX | Serial communication |
GPIO 3 | RX | Serial communication |
GPIO 4 | Camera SDA | |
GPIO 12 | LED illumination | Control for onboard LED |
GPIO 13 | Red LED | Status indicator |
GPIO 14 | Camera SCL | |
GPIO 16 | Camera reset |
Initial Setup
Development Environment Configuration
Install Arduino IDE:
Download and install the latest version from arduino.cc
Add ESP32 board manager URL in Preferences:
https://dl.espressif.com/dl/package_esp32_index.json
Install ESP32 board support via Tools → Board → Boards Manager
Required Libraries:
ESP32 Arduino Core
ESP32 Camera library
For advanced applications: TensorFlow Lite, Firebase ESP32 Client
Board Selection:
Select "AI Thinker ESP32-CAM" from the boards menu
Hardware Connection for Programming
When programming the ESP32-CAM, you'll need to connect it to your computer using an FTDI programmer with the following connections:
ESP32-CAM | FTDI Programmer |
---|---|
5V | VCC (5V) |
GND | GND |
U0R (RX) | TX |
U0T (TX) | RX |
GPIO 0 | GND (during upload only) |
Important: GPIO 0 must be connected to GND during programming to enter bootloader mode. Disconnect after programming.
First Test: Camera Web Server
Open Arduino IDE and load the example:
File → Examples → ESP32 → Camera → CameraWebServer
Configure your Wi-Fi credentials in the sketch:
const char* ssid = "YOUR_WIFI_SSID"; const char* password = "YOUR_WIFI_PASSWORD";
Ensure the camera model is correctly set (uncomment the appropriate line):
#define CAMERA_MODEL_AI_THINKER // ESP32-CAM
Connect GPIO 0 to GND, then press the reset button on the ESP32-CAM.
Upload the sketch. Once complete, disconnect GPIO 0 from GND and press reset again.
Open the Serial Monitor (set to 115200 baud) to find the assigned IP address.
Visit the IP address in a web browser to access the camera web interface.
Power Optimization
The ESP32-CAM can consume significant power, especially with active Wi-Fi and camera operations. Here are strategies to optimize battery life:
Deep Sleep Implementation
#include "esp_camera.h"
#include "esp_timer.h"
#include "esp_sleep.h"
// Time to sleep (in seconds)
#define TIME_TO_SLEEP 60
void enterDeepSleep() {
// Disable camera
esp_camera_deinit();
// Configure wake-up source
esp_sleep_enable_timer_wakeup(TIME_TO_SLEEP * 1000000);
// Enter deep sleep
Serial.println("Going to deep sleep now");
esp_deep_sleep_start();
}
Power Reduction Techniques
Wi-Fi Duty Cycling:
WiFi.mode(WIFI_OFF); // Turn off Wi-Fi when not needed
Camera Power Management:
// Power down camera sensor sensor_t * s = esp_camera_sensor_get(); s->set_framesize(s, FRAMESIZE_QVGA); // Lower resolution s->set_hmirror(s, 0); // Disable features s->set_vflip(s, 0);
Adjust CPU Frequency:
setCpuFrequencyMhz(80); // Reduce from 240MHz to 80MHz
Image Capture and Processing
Basic Image Capture
#include "esp_camera.h"
camera_fb_t * fb = NULL;
void captureImage() {
// Capture frame
fb = esp_camera_fb_get();
if (!fb) {
Serial.println("Camera capture failed");
return;
}
// Process image data in fb->buf, size fb->len
// Return frame buffer when done
esp_camera_fb_return(fb);
}
Saving Images to MicroSD Card
#include "SD_MMC.h"
#include "FS.h"
void saveImageToSD() {
// Capture image
camera_fb_t * fb = esp_camera_fb_get();
if (!fb) {
Serial.println("Camera capture failed");
return;
}
// Initialize microSD card
if (!SD_MMC.begin()) {
Serial.println("SD Card Mount Failed");
esp_camera_fb_return(fb);
return;
}
// Create file path with timestamp
String path = "/image_" + String(millis()) + ".jpg";
// Save image
File file = SD_MMC.open(path.c_str(), FILE_WRITE);
if (!file) {
Serial.println("Failed to open file for writing");
} else {
file.write(fb->buf, fb->len);
Serial.printf("Saved image to %s\n", path.c_str());
}
file.close();
SD_MMC.end();
esp_camera_fb_return(fb);
}
Basic Image Processing
For simple processing, we can implement brightness adjustment and grayscale conversion directly:
void adjustBrightness(uint8_t* buffer, size_t length, int factor) {
for (size_t i = 0; i < length; i++) {
// Apply brightness factor with limits
int newValue = buffer[i] + factor;
buffer[i] = constrain(newValue, 0, 255);
}
}
void convertToGrayscale(camera_fb_t* fb) {
if (fb->format != PIXFORMAT_RGB565) {
return; // Only works with RGB565 format
}
uint8_t* buf = fb->buf;
for (size_t i = 0; i < fb->len; i += 2) {
uint16_t pixel = (buf[i+1] << 8) | buf[i];
// Extract RGB components
uint8_t r = (pixel >> 11) & 0x1F;
uint8_t g = (pixel >> 5) & 0x3F;
uint8_t b = pixel & 0x1F;
// Convert to grayscale
uint8_t gray = (r * 77 + g * 151 + b * 28) >> 8;
// Pack back into RGB565
uint16_t grayPixel = (gray >> 3) << 11 | (gray >> 2) << 5 | (gray >> 3);
buf[i] = grayPixel & 0xFF;
buf[i+1] = (grayPixel >> 8) & 0xFF;
}
}
Advanced Applications
Motion Detection
Motion detection can be implemented by comparing consecutive frames:
#define WIDTH 320
#define HEIGHT 240
#define BLOCK_SIZE 16
#define MOTION_THRESHOLD 20
uint8_t prev_frame[WIDTH * HEIGHT];
bool first_frame = true;
bool detectMotion() {
camera_fb_t * fb = esp_camera_fb_get();
if (!fb) return false;
if (fb->format != PIXFORMAT_GRAYSCALE) {
// Convert to grayscale if needed
// Implementation depends on input format
}
if (first_frame) {
memcpy(prev_frame, fb->buf, WIDTH * HEIGHT);
first_frame = false;
esp_camera_fb_return(fb);
return false;
}
// Compare blocks for motion
int changed_blocks = 0;
for (int y = 0; y < HEIGHT; y += BLOCK_SIZE) {
for (int x = 0; x < WIDTH; x += BLOCK_SIZE) {
int diff_sum = 0;
// Compare pixels in block
for (int j = 0; j < BLOCK_SIZE; j++) {
for (int i = 0; i < BLOCK_SIZE; i++) {
int pos = (y + j) * WIDTH + (x + i);
diff_sum += abs(fb->buf[pos] - prev_frame[pos]);
}
}
// Calculate average difference
int avg_diff = diff_sum / (BLOCK_SIZE * BLOCK_SIZE);
if (avg_diff > MOTION_THRESHOLD) {
changed_blocks++;
}
}
}
// Update previous frame
memcpy(prev_frame, fb->buf, WIDTH * HEIGHT);
esp_camera_fb_return(fb);
return (changed_blocks > (HEIGHT * WIDTH) / (BLOCK_SIZE * BLOCK_SIZE * 10));
}
Face Detection
The ESP32-CAM can perform simple face detection using the built-in functionality:
#include "fd_forward.h"
mtmn_config_t mtmn_config = {0};
void setupFaceDetection() {
mtmn_config.type = FAST;
mtmn_config.min_face = 80;
mtmn_config.pyramid = 0.707;
mtmn_config.pyramid_times = 4;
mtmn_config.p_threshold.score = 0.6;
mtmn_config.p_threshold.nms = 0.7;
mtmn_config.p_threshold.candidate_number = 20;
mtmn_config.r_threshold.score = 0.7;
mtmn_config.r_threshold.nms = 0.7;
mtmn_config.r_threshold.candidate_number = 10;
mtmn_config.o_threshold.score = 0.7;
mtmn_config.o_threshold.nms = 0.7;
mtmn_config.o_threshold.candidate_number = 1;
}
bool detectFace() {
camera_fb_t * fb = esp_camera_fb_get();
if (!fb) return false;
// Run face detection algorithm
dl_matrix3du_t *image_matrix = dl_matrix3du_alloc(1, fb->width, fb->height, 3);
if (!image_matrix) {
esp_camera_fb_return(fb);
return false;
}
// Convert frame to RGB format for detection
fmt2rgb888(fb->buf, fb->len, fb->format, image_matrix->item);
// Detect faces
box_array_t *boxes = face_detect(image_matrix, &mtmn_config);
// Clean up
dl_matrix3du_free(image_matrix);
esp_camera_fb_return(fb);
// Return true if faces were detected
if (boxes) {
// Process face coordinates if needed: boxes->box[i].box_p[0-3]
free(boxes);
return true;
}
return false;
}
Networking and Cloud Integration
Implementing HTTP Server for Remote Viewing
#include "ESPAsyncWebServer.h"
AsyncWebServer server(80);
void setupWebServer() {
// Route for root
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
String html = "<html><body>";
html += "<h1>ESP32-CAM Control</h1>";
html += "<img src='/capture' id='cam'>";
html += "<script>setInterval(function(){";
html += "document.getElementById('cam').src='/capture?'+new Date().getTime();";
html += "}, 1000);</script></body></html>";
request->send(200, "text/html", html);
});
// Route for capturing image
server.on("/capture", HTTP_GET, [](AsyncWebServerRequest *request){
camera_fb_t * fb = esp_camera_fb_get();
if (!fb) {
request->send(500, "text/plain", "Camera capture failed");
return;
}
request->send_P(200, "image/jpeg", fb->buf, fb->len);
esp_camera_fb_return(fb);
});
// Start server
server.begin();
}
MQTT Integration for IoT Applications
#include <PubSubClient.h>
#include <WiFi.h>
WiFiClient espClient;
PubSubClient client(espClient);
void setupMQTT() {
client.setServer("your-mqtt-broker.com", 1883);
client.setCallback(callback);
}
void reconnectMQTT() {
while (!client.connected()) {
Serial.println("Connecting to MQTT...");
if (client.connect("ESP32CAM", "mqtt_user", "mqtt_password")) {
Serial.println("Connected");
client.subscribe("esp32cam/control");
} else {
Serial.print("Failed, rc=");
Serial.print(client.state());
Serial.println(" Retrying in 5 seconds");
delay(5000);
}
}
}
void callback(char* topic, byte* payload, unsigned int length) {
String message;
for (int i = 0; i < length; i++) {
message += (char)payload[i];
}
if (message == "capture") {
camera_fb_t * fb = esp_camera_fb_get();
if (fb) {
// Convert image to base64 if needed
// Publish image data
client.publish("esp32cam/image", fb->buf, fb->len);
esp_camera_fb_return(fb);
}
}
}
Google Firebase Integration
#include "FirebaseESP32.h"
#define FIREBASE_HOST "your-project.firebaseio.com"
#define FIREBASE_AUTH "your-firebase-auth-token"
FirebaseData firebaseData;
void setupFirebase() {
Firebase.begin(FIREBASE_HOST, FIREBASE_AUTH);
Firebase.reconnectWiFi(true);
}
void uploadImageToFirebase() {
camera_fb_t * fb = esp_camera_fb_get();
if (!fb) {
Serial.println("Camera capture failed");
return;
}
String path = "/images/" + String(millis());
if (Firebase.setBlob(firebaseData, path, fb->buf, fb->len)) {
Serial.println("Image uploaded successfully");
Serial.println("URL: " + firebaseData.dataPath());
} else {
Serial.println("Failed to upload image");
Serial.println(firebaseData.errorReason());
}
esp_camera_fb_return(fb);
}
Common Challenges and Solutions
Connectivity Issues
Weak Wi-Fi Signal
Add an external antenna (some ESP32-CAM models have an IPEX connector)
Reduce distance to router or use a Wi-Fi repeater
Implement a mesh network with multiple ESP32 devices
Unstable Connection
Add proper power filtering (add 100μF and 0.1μF capacitors between VCC and GND)
Implement reconnection logic:
void ensureWiFiConnected() { if (WiFi.status() != WL_CONNECTED) { Serial.println("Reconnecting to WiFi..."); WiFi.reconnect(); int attempts = 0; while (WiFi.status() != WL_CONNECTED && attempts < 20) { delay(500); Serial.print("."); attempts++; } }}
Memory Management
The ESP32-CAM has limited memory, which can cause crashes when processing large images:
Use PSRAM efficiently:
camera_config_t config; config.frame_size = FRAMESIZE_SVGA; config.jpeg_quality = 12; // 0-63, lower is higher quality config.fb_count = 2; config.fb_location = CAMERA_FB_IN_PSRAM; // Use PSRAM for frame buffer
Process images in chunks rather than loading entire images into memory.
Implement memory monitoring:
void checkMemory() { Serial.printf("Free heap: %d, PSRAM: %d\n", ESP.getFreeHeap(), ESP.getFreePsram()); }
Camera Quality Optimization
Adjust camera settings:
sensor_t * s = esp_camera_sensor_get(); s->set_brightness(s, 1); // -2 to 2 s->set_contrast(s, 1); // -2 to 2 s->set_saturation(s, 0); // -2 to 2 s->set_special_effect(s, 0); // 0 = No Effect, 1 = Negative, 2 = Grayscale s->set_whitebal(s, 1); // 0 = disable, 1 = enable s->set_awb_gain(s, 1); // 0 = disable, 1 = enable s->set_wb_mode(s, 0); // 0 to 4 - various WB modes s->set_exposure_ctrl(s, 1); // 0 = disable, 1 = enable s->set_aec2(s, 0); // 0 = disable, 1 = enable s->set_gain_ctrl(s, 1); // 0 = disable, 1 = enable s->set_agc_gain(s, 0); // 0 to 30 s->set_gainceiling(s, (gainceiling_t)0); // 0 to 6 s->set_bpc(s, 0); // 0 = disable, 1 = enable s->set_wpc(s, 1); // 0 = disable, 1 = enable s->set_raw_gma(s, 1); // 0 = disable, 1 = enable s->set_lenc(s, 1); // 0 = disable, 1 = enable s->set_hmirror(s, 0); // 0 = disable, 1 = enable s->set_vflip(s, 0); // 0 = disable, 1 = enable s->set_dcw(s, 1); // 0 = disable, 1 = enable
Lighting considerations:
Use the built-in LED for consistent lighting:
// Control flash LEDconst int flashPin = 4;pinMode(flashPin, OUTPUT);digitalWrite(flashPin, HIGH); // Turn on LEDdelay(100); // Give time for light to stabilize// Capture imagedigitalWrite(flashPin, LOW); // Turn off LED
For outdoor applications, consider adding a light shield to prevent direct sunlight on the lens.
Project Ideas and Use Cases
Home Security System
Build a complete home security system with motion detection, cloud notification, and remote viewing:
Features:
Motion-activated recording
Cloud storage of captured images
Push notifications to mobile devices
Live streaming via web interface
Implementation Approach:
Use deep sleep mode for battery operation
Wake on PIR sensor trigger
Capture and upload images when motion is detected
Send push notifications via Firebase Cloud Messaging
Plant Monitoring System
Monitor plant health and automate watering:
Features:
Time-lapse plant growth photography
Color analysis for plant health assessment
Automated watering system integration
Climate data correlation
Implementation:
Scheduled image capture
Image analysis for leaf color and growth metrics
Integration with soil moisture sensors
Automated irrigation control
Wildlife Camera Trap
Create a low-cost wildlife monitoring solution:
Features:
Motion-triggered image capture
Long battery life (weeks/months)
Local storage with periodic uploads
Animal recognition capabilities
Implementation:
Deep sleep with PIR or radar sensor wake-up
Weatherproof housing design
Solar panel integration for extended operation
TensorFlow Lite for simple species classification
Conclusion
The ESP32-CAM represents a significant advancement in accessible computer vision, enabling makers, hobbyists, and professionals to implement vision capabilities in projects at an unprecedented price point. While it has limitations in processing power and image quality compared to more expensive solutions, its combination of connectivity, programmability, and low cost makes it ideal for many edge vision applications.
By following this implementation guide, you should now have a solid foundation for integrating ESP32-CAM into your own projects. As the ESP32 ecosystem continues to evolve, we can expect even more capabilities and optimizations that will further enhance this already impressive platform.
Additional Resources
Article Info
Engage
Table of Contents
- Hardware Overview
- Initial Setup
- Development Environment Configuration
- Hardware Connection for Programming
- First Test: Camera Web Server
- Power Optimization
- Image Capture and Processing
- Advanced Applications
- Networking and Cloud Integration
- Implementing HTTP Server for Remote Viewing
- MQTT Integration for IoT Applications
- Google Firebase Integration
- Common Challenges and Solutions
- Project Ideas and Use Cases
- Conclusion
- Additional Resources