Understanding Real-Time Operating Systems (RTOS): From Basics to Advanced Applications
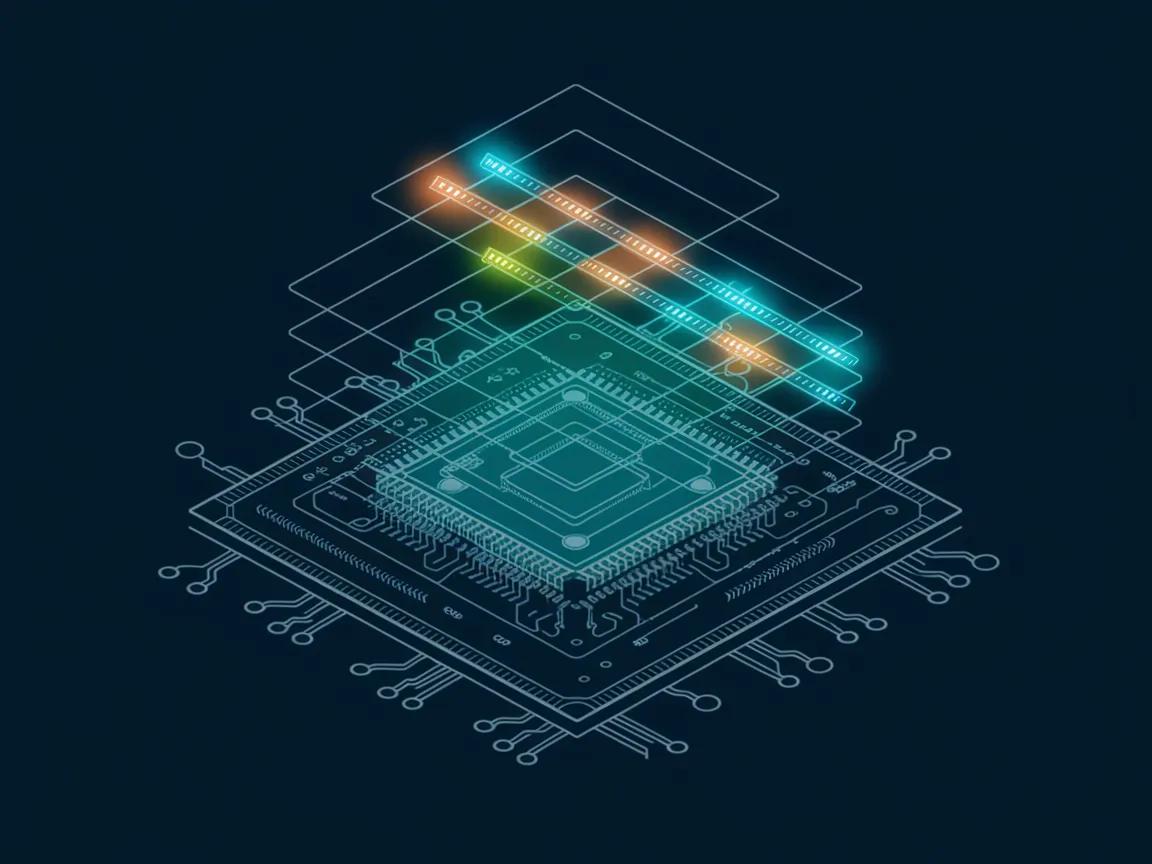
Article
Abstract
Real-Time Operating Systems (RTOS) form the backbone of modern embedded systems where timing predictability is critical. This comprehensive article explores the architecture, implementation methodologies, and applications of RTOS across various industries, with a particular focus on aerospace, automotive, and medical sectors. We examine how RTOS differs from general-purpose operating systems, discuss key features like deterministic behavior and low latency, and analyze real-world deployment challenges and solutions. The article concludes with an exploration of emerging trends, including the integration of AI capabilities within real-time environments and the evolution of RTOS for IoT and edge computing applications.
1. Introduction
In our increasingly connected world, many systems require not just correct computational results, but results delivered within strict timing constraints. A flight control system, medical ventilator, or automotive anti-lock brake system must respond to inputs within guaranteed timeframes—mere milliseconds can mean the difference between safety and catastrophe.
Real-Time Operating Systems (RTOS) were developed to meet these precise timing requirements. Unlike general-purpose operating systems like Windows or Linux that optimize for average-case performance and user experience, RTOS prioritize deterministic behavior and predictable response times.
The need for RTOS extends far beyond traditional embedded systems. Today, they power critical infrastructure in telecommunications, industrial automation, aerospace, healthcare, and automotive applications. As embedded devices become more powerful and connected, RTOS continue to evolve, balancing the demands of real-time performance with expanded functionality.
This article provides a comprehensive examination of RTOS—their architecture, implementation methodologies, real-world applications, and future trends. Whether you're an embedded systems engineer, a software architect, or simply interested in understanding the technology running mission-critical systems, this guide offers valuable insights into the world of real-time computing.
2. Historical Context and Evolution
Origins of Real-Time Computing
The concept of real-time computing emerged in the 1950s with early military and aerospace applications. The Apollo Guidance Computer (AGC) developed in the 1960s for NASA's Apollo program is often considered one of the first operational real-time computing systems, though it used specialized software rather than a commercial RTOS.
First Commercial RTOS
The 1970s saw the emergence of commercial RTOS products:
VRTX (Versatile Real-Time Executive) was created by Hunter & Ready in 1980 and later became a flagship product at Microtec Research.
VxWorks, developed by Wind River Systems in 1987, became one of the most successful commercial RTOS, particularly in aerospace and defense applications.
QNX, released in 1982, pioneered microkernel architecture for real-time systems and later became prominent in automotive and industrial control.
Evolution and Standardization
The 1990s brought significant developments in RTOS standardization:
The POSIX (Portable Operating System Interface) standards incorporated real-time extensions (POSIX.1b) to facilitate software portability across different RTOS.
The OSEK/VDX standard emerged in the automotive industry, providing a common RTOS specification for vehicle electronic control units.
ITRON (Industrial TRON) became the dominant RTOS specification in Japan, particularly for consumer electronics.
Modern RTOS Landscape
Today's RTOS landscape includes a mix of proprietary and open-source solutions:
Commercial RTOS: VxWorks (Wind River), QNX (BlackBerry), Integrity (Green Hills Software), and ThreadX (Microsoft) dominate mission-critical applications where certification and support are essential.
Open-Source RTOS: FreeRTOS (now part of Amazon Web Services), Zephyr (Linux Foundation), and RIOT have gained significant traction, especially for IoT and cost-sensitive applications.
Hybrid Approaches: RTLinux and Xenomai add real-time capabilities to Linux, balancing deterministic performance with the rich functionality of a general-purpose OS.
As embedded systems have become more powerful, the line between RTOS and general-purpose operating systems has blurred, with many solutions now supporting rich networking, graphics, and file system capabilities while maintaining real-time guarantees.
3. Technical Deep-Dive: RTOS Architecture
Core Components
An RTOS consists of several key components:
Kernel: The central component managing hardware resources, providing services to applications, and implementing the scheduling algorithm.
Task Manager: Handles task creation, deletion, and state transitions.
Scheduler: Determines which task runs next based on priorities and timing requirements.
Interrupt Handler: Manages hardware and software interrupts to ensure timely response to external events.
Memory Manager: Allocates and deallocates memory, often with deterministic algorithms to avoid unpredictable delays.
Timer Services: Provides high-resolution timing facilities for tasks and timeouts.
Inter-Process Communication (IPC): Mechanisms for tasks to exchange data and synchronize execution.
Task Scheduling Algorithms
RTOS employ various scheduling algorithms to ensure predictable execution:
Fixed Priority Preemptive Scheduling: Tasks are assigned static priorities, and a higher-priority task can preempt a lower-priority one. This is the most common approach in commercial RTOS.
Rate Monotonic Scheduling (RMS): A static scheduling algorithm where priorities are assigned based on task periods—shorter periods receive higher priorities.
Earliest Deadline First (EDF): A dynamic scheduling algorithm where priorities change based on which task has the nearest deadline.
Time-Triggered Architecture (TTA): Tasks execute according to a predefined time schedule, eliminating runtime scheduling decisions and improving determinism.
Task Management
RTOS support various task states and transitions:
Ready: The task is ready to execute but waiting for the scheduler to allocate CPU time.
Running: The task is currently executing on the CPU.
Blocked: The task is waiting for a resource or event (e.g., I/O completion, a semaphore, or timeout).
Suspended: The task is temporarily inactive and won't be scheduled until explicitly resumed.
Terminated: The task has completed execution or has been forcibly terminated.
Here's an example of task creation in FreeRTOS:
void vTaskFunction(void *pvParameters) {
for (;;) {
// Task implementation
vTaskDelay(pdMS_TO_TICKS(100)); // Sleep for 100ms
}
}
int main(void) {
// Create a task with priority 1 and 2KB stack
xTaskCreate(
vTaskFunction, // Function that implements the task
"ExampleTask", // Text name for the task
2048, // Stack size in words, not bytes
NULL, // Parameter passed into the task
1, // Priority at which the task is created
NULL // Used to pass out the created task's handle
);
// Start the scheduler
vTaskStartScheduler();
// Will only reach here if there's insufficient heap for the idle task
for (;;);
}
Memory Management
RTOS memory management differs from general-purpose OS approaches:
Static Allocation: Many RTOS applications pre-allocate all required memory at initialization to avoid unpredictable allocation delays during operation.
Memory Pools: Fixed-size blocks are pre-allocated for specific purposes, ensuring constant-time allocation.
Deterministic Allocation: When dynamic allocation is necessary, RTOS use algorithms with bounded execution times.
Memory Protection: Modern RTOS utilize hardware Memory Protection Units (MPU) or Memory Management Units (MMU) to isolate tasks and prevent memory corruption.
Inter-Process Communication
RTOS provide various IPC mechanisms with deterministic behavior:
Semaphores: Control access to shared resources, available in binary (mutex) and counting forms.
Message Queues: Allow tasks to exchange data in a producer-consumer pattern with priority inheritance.
Event Flags: Enable tasks to signal conditions without passing data.
Pipes and Mailboxes: Support larger data transfers between tasks.
Shared Memory: Allows direct access to common data areas, often with synchronization mechanisms.
Example of message queue usage in VxWorks:
MSG_Q_ID msgQId;
char message[100];
void senderTask(void *arg) {
while (1) {
// Prepare message
sprintf(message, "Sensor reading: %d", readSensor());
// Send message with normal priority (1), waiting indefinitely
if (msgQSend(msgQId, message, strlen(message)+1, WAIT_FOREVER, MSG_PRI_NORMAL) == ERROR) {
printf("Error sending message\n");
}
// Wait before next reading
taskDelay(sysClkRateGet() / 10); // 100ms delay
}
}
void receiverTask(void *arg) {
char buffer[100];
while (1) {
// Receive message, waiting indefinitely
if (msgQReceive(msgQId, buffer, sizeof(buffer), WAIT_FOREVER) == ERROR) {
printf("Error receiving message\n");
} else {
printf("Received: %s\n", buffer);
processData(buffer);
}
}
}
int initTasks(void) {
// Create message queue with 10 messages max, each 100 bytes max
msgQId = msgQCreate(10, 100, MSG_Q_FIFO);
if (msgQId == NULL) {
printf("Error creating message queue\n");
return ERROR;
}
// Create tasks
if (taskSpawn("tSender", 100, 0, 2000, (FUNCPTR)senderTask, 0,0,0,0,0,0,0,0,0,0) == ERROR) {
printf("Error creating sender task\n");
return ERROR;
}
if (taskSpawn("tReceiver", 90, 0, 2000, (FUNCPTR)receiverTask, 0,0,0,0,0,0,0,0,0,0) == ERROR) {
printf("Error creating receiver task\n");
return ERROR;
}
return OK;
}
Real-Time Clock and Timer Services
Precise timing is fundamental to RTOS operation:
System Tick: Most RTOS use a periodic interrupt (system tick) as the fundamental timing unit, typically configured between 1ms and 10ms.
High-Resolution Timers: For applications requiring finer timing control, many RTOS provide microsecond or nanosecond resolution timers.
Certification Needs: Safety certification (DO-178C for aerospace, ISO 26262 for automotive, IEC 62304 for medical).
Watchdog Timers: Monitor system behavior and take corrective action if a task fails to respond within a specified time.
Time-based Scheduling: Services to delay tasks, wake them at specific times, or execute them periodically.
4. Implementation Methodologies
RTOS Selection Criteria
Selecting the right RTOS involves evaluating:
Performance Requirements: Interrupt latency, context switch time, and memory footprint.
Hardware Compatibility: Processor architecture support, device driver availability, and BSP (Board Support Package) quality.
Certification Needs: Safety certification (DO-178C for aerospace, ISO 26262 for automotive, IEC 62304 for medical).
Development Environment: Quality of tools, debuggers, and middleware.
Licensing and Costs: Commercial vs. open-source considerations, royalty structures.
Ecosystem and Support: Availability of training, technical support, and third-party integrations.
Development Process
Developing RTOS-based applications typically follows these steps:
System Requirements Analysis: Define timing constraints, resource requirements, and interaction patterns..
Task Decomposition: Divide the application into tasks with clear responsibilities and priorities.
Resource Allocation: Plan memory usage, peripheral access, and shared resource management.
Implementation: Develop task code, IPC mechanisms, and system initialization.
Integration and Testing: Verify timing behavior, resource usage, and functionality.
Performance Optimization: Tune priorities, reduce latency, and minimize resource contention.
Optimization Techniques
Optimizing RTOS-based applications involves:
Priority Assignment: Assigning appropriate priorities based on task criticality and deadline requirements.
Minimizing Shared Resources: Reducing potential for priority inversion and blocking.
Interrupt Handling Optimization: Keeping ISRs (Interrupt Service Routines) short and deferring work to tasks when possible.
Memory Access Patterns: Organizing data structures to minimize cache misses and memory contention.
Stack Size Tuning: Allocating sufficient but not excessive stack space to each task.
Common Pitfalls
Developers commonly encounter these challenges:
Priority Inversion: Occurs when a high-priority task is indirectly blocked by a low-priority task. Solved through priority inheritance or priority ceiling protocols.
Deadlocks: Tasks permanently blocking each other due to circular resource dependencies. Avoided through careful resource allocation ordering.
Unbound Priority Inversion: A variant of priority inversion without time limits. Prevented using timeout mechanisms for all blocking operations.
Race Conditions: Improper synchronization leading to data corruption. Addressed with proper mutex usage.
Stack Overflow: Tasks exceeding their allocated stack space. Detected through stack monitoring and addressed by increasing allocation or optimizing recursion.
Case Study: VxWorks in Aerospace Applications
Wind River's VxWorks has been a dominant RTOS in aerospace applications, including NASA's Mars rovers. Key implementation aspects include:
Hardware Abstraction : Using the Board Support Package (BSP) to isolate hardware-specific code from application logic.
Redundancy Management: Tasks permanently blocking each other due to circular resource dependencies. Avoided through careful resource allocation ordering.
Health Monitoring: Continuous system monitoring with watchdog timers and error detection.
Resource Partitioning: Isolating critical components from less critical ones to prevent interference.
Stack Overflow: Generating documentation and test results to satisfy DO-178C certification requirements.
Following code example demonstrates a simplified flight control system task in VxWorks:
#include <vxWorks.h>
#include <taskLib.h>
#include <sysLib.h>
#include <semLib.h>
#define CONTROL_LOOP_PERIOD_MS 10 // 10ms control loop
#define SENSOR_SAMPLING_PERIOD_MS 5 // 5ms sensor sampling
// Semaphores for synchronization
SEM_ID sensorDataSem;
SEM_ID actuatorCmdSem;
// Shared data structures
typedef struct {
float altitude;
float attitude[3]; // roll, pitch, yaw
float acceleration[3]; // x, y, z
uint32_t timestamp;
} SensorData;
typedef struct {
float thrustCommand;
float controlSurfaces[4]; // aileron, elevator, rudder, flaps
uint32_t timestamp;
} ActuatorCommands;
SensorData sensorData;
ActuatorCommands actuatorCommands;
// Sensor sampling task - higher priority
void sensorTask(void) {
struct timespec startTime, endTime, sleepTime;
int loopCount = 0;
while (1) {
// Record start time
clock_gettime(CLOCK_REALTIME, &startTime);
// Sample sensors
readInertialSensors(&sensorData.attitude[0], &sensorData.acceleration[0]);
readAltimeter(&sensorData.altitude);
sensorData.timestamp = startTime.tv_sec * 1000 + startTime.tv_nsec / 1000000;
// Signal that new sensor data is available
semGive(sensorDataSem);
// Log timing every 1000 iterations
if (++loopCount % 1000 == 0) {
clock_gettime(CLOCK_REALTIME, &endTime);
long processingTime = (endTime.tv_sec - startTime.tv_sec) * 1000000 +
(endTime.tv_nsec - startTime.tv_nsec) / 1000;
printf("Sensor task processing time: %ld microseconds\n", processingTime);
}
// Calculate sleep time for precise periodic execution
clock_gettime(CLOCK_REALTIME, &endTime);
sleepTime.tv_sec = 0;
sleepTime.tv_nsec = SENSOR_SAMPLING_PERIOD_MS * 1000000 -
((endTime.tv_sec - startTime.tv_sec) * 1000000000 +
(endTime.tv_nsec - startTime.tv_nsec));
if (sleepTime.tv_nsec > 0) {
nanosleep(&sleepTime, NULL);
} else {
printf("WARNING: Sensor task exceeded period!\n");
}
}
}
// Control law execution task
void controlTask(void) {
struct timespec startTime, endTime, sleepTime;
int loopCount = 0;
SensorData localSensorData;
while (1) {
// Record start time
clock_gettime(CLOCK_REALTIME, &startTime);
// Wait for sensor data
if (semTake(sensorDataSem, CONTROL_LOOP_PERIOD_MS) == ERROR) {
printf("WARNING: Sensor data not available within timeout!\n");
continue;
}
// Copy sensor data to local structure to minimize critical section
memcpy(&localSensorData, &sensorData, sizeof(SensorData));
// Execute control laws
executeAttitudeControl(localSensorData.attitude,
&actuatorCommands.controlSurfaces[0]);
executeAltitudeControl(localSensorData.altitude,
&actuatorCommands.thrustCommand);
actuatorCommands.timestamp = startTime.tv_sec * 1000 + startTime.tv_nsec / 1000000;
// Signal that new actuator commands are available
semGive(actuatorCmdSem);
// Log timing every 1000 iterations
if (++loopCount % 1000 == 0) {
clock_gettime(CLOCK_REALTIME, &endTime);
long processingTime = (endTime.tv_sec - startTime.tv_sec) * 1000000 +
(endTime.tv_nsec - startTime.tv_nsec) / 1000;
printf("Control task processing time: %ld microseconds\n", processingTime);
}
// Calculate sleep time for precise periodic execution
clock_gettime(CLOCK_REALTIME, &endTime);
sleepTime.tv_sec = 0;
sleepTime.tv_nsec = CONTROL_LOOP_PERIOD_MS * 1000000 -
((endTime.tv_sec - startTime.tv_sec) * 1000000000 +
(endTime.tv_nsec - startTime.tv_nsec));
if (sleepTime.tv_nsec > 0) {
nanosleep(&sleepTime, NULL);
} else {
printf("WARNING: Control task exceeded period!\n");
}
}
}
// Actuator output task - lower priority
void actuatorTask(void) {
ActuatorCommands localActuatorCommands;
while (1) {
// Wait for actuator commands (wait forever)
semTake(actuatorCmdSem, WAIT_FOREVER);
// Copy commands to local structure to minimize critical section
memcpy(&localActuatorCommands, &actuatorCommands, sizeof(ActuatorCommands));
// Send commands to hardware
setControlSurfaces(localActuatorCommands.controlSurfaces);
setThrustLevel(localActuatorCommands.thrustCommand);
// Calculate and log command latency
struct timespec currentTime;
clock_gettime(CLOCK_REALTIME, ¤tTime);
uint32_t currentTimeMs = currentTime.tv_sec * 1000 + currentTime.tv_nsec / 1000000;
uint32_t latency = currentTimeMs - localActuatorCommands.timestamp;
if (latency > 5) { // Log if latency exceeds 5ms
printf("WARNING: Actuator command latency: %u ms\n", latency);
}
}
}
// Initialize the system
int initFlightControlSystem(void) {
// Create semaphores
sensorDataSem = semBCreate(SEM_Q_PRIORITY, SEM_EMPTY);
actuatorCmdSem = semBCreate(SEM_Q_PRIORITY, SEM_EMPTY);
if (sensorDataSem == NULL || actuatorCmdSem == NULL) {
printf("Failed to create semaphores\n");
return ERROR;
}
// Create tasks with appropriate priorities (higher number = higher priority)
if (taskSpawn("tSensor", 110, 0, 8192, (FUNCPTR)sensorTask,
0,0,0,0,0,0,0,0,0,0) == ERROR) {
printf("Failed to create sensor task\n");
return ERROR;
}
if (taskSpawn("tControl", 100, 0, 8192, (FUNCPTR)controlTask,
0,0,0,0,0,0,0,0,0,0) == ERROR) {
printf("Failed to create control task\n");
return ERROR;
}
if (taskSpawn("tActuator", 90, 0, 8192, (FUNCPTR)actuatorTask,
0,0,0,0,0,0,0,0,0,0) == ERROR) {
printf("Failed to create actuator task\n");
return ERROR;
}
printf("Flight control system initialized\n");
return OK;
}
5. Real-World Applications
Aerospace and Defense
RTOS are essential in aerospace applications:
Flight Control Systems: Managing control surfaces with strict timing requirements.
Avionics: Integrating navigation, communication, and monitoring systems.
Space Exploration: Powering satellites and planetary rovers with autonomous operation capabilities.
Defense Systems: Operating in radar, missile guidance, and electronic warfare applications.
VxWorks, LynxOS-178, and Integrity-178 dominate this sector due to their DO-178B/C certification for safety-critical aviation software.
Automotive
Modern vehicles contain numerous RTOS instances:
Engine Control Units (ECUs): Managing fuel injection, ignition timing, and emissions.
Advanced Driver Assistance Systems (ADAS): Processing sensor data for features like adaptive cruise control and lane keeping assistance.
Autonomous Driving Systems: Handling real-time decision making and vehicle control.
Infotainment Systems: Combining real-time vehicle data with user interface applications.
AUTOSAR (AUTomotive Open System ARchitecture) has standardized automotive software architecture, with QNX, INTEGRITY, and various OSEK/VDX-compliant RTOS being widely adopted.
Industrial Automation
Industrial applications include:
Programmable Logic Controllers (PLCs): Controlling manufacturing processes with deterministic timing.
Robotics: Coordinating motion control, vision systems, and safety monitoring.
Process Control: Managing continuous manufacturing processes in industries like chemical, oil and gas, and pharmaceuticals.
SCADA System: Supervising distributed control systems across industrial facilities.
VxWorks, QNX, and industrial-grade Linux with real-time extensions (Xenomai, PREEMPT_RT) are common in these applications.
Medical Devices
Life-critical medical equipment relies on RTOS:
Patient Monitoring System: Processing vital signs with guaranteed response times.
Infusion Pumps: Delivering precise medication doses with strict timing requirements.
Ventilators: Controlling breathing cycles with millisecond precision.
Surgical Robots: Coordinating precise movements with sensor feedback.
QNX, VxWorks, and INTEGRITY are frequently used in devices requiring IEC 62304 medical software certification.
Telecommunications
Telecom infrastructure depends on RTOS for:
Base Stations: Processing cellular signals with strict timing requirements.
Network Switches and Routers: Handling packet processing with deterministic latency.
VoIP Systems: Managing real-time voice data transmission.
Satellite Communications: Coordinating signal processing and network management.
Carrier-grade Linux with real-time extensions and specialized RTOS like VxWorks Telecom Platform are common in these applications.
Consumer Electronics
Even consumer devices benefit from RTOS capabilities:
Digital Cameras: Managing image processing pipelines and autofocus systems.
Premium Audio Equipment: Processing audio signals with low latency.
Smart Home Devices: Coordinating sensor inputs and control outputs.
Wearable Health Monitors: Processing biometric data with energy efficiency.
FreeRTOS, Zephyr, and ThreadX are popular in these applications due to their smaller footprint and lower licensing costs.
6. Challenges and Solutions
Real-Time Performance Validation
Ensuring deterministic behavior involves:
Timing Analysis: Using static and dynamic methods to verify worst-case execution times.
Scheduling Analysis: Validating that all tasks meet their deadlines under worst-case scenarios.
Load Testing: Subjecting the system to maximum expected loads to verify responsiveness.
Long-Term Testing: Running systems continuously to detect timing anomalies that appear rarely.
Tools like RapiTime, SymTA/S, and Tracealyzer help analyze and visualize real-time behavior.
Safety and Certification
Critical applications require formal certification:
DO-178C: For airborne systems, requiring evidence of requirements traceability, code coverage, and formal verification.
ISO 26262: For automotive applications, focusing on functional safety and risk assessment.
IEC 61508: General functional safety standard for electrical/electronic/programmable electronic safety-related systems.
IEC 62304: For medical device software, ensuring risk management throughout the development lifecycle.
RTOS vendors often provide certification evidence packages to help customers through these processes.
Security Considerations
Modern RTOS must address cybersecurity concerns:
Secure Boot: Ensuring only authenticated software can run on the device.
Memory Protection: Preventing one task from corrupting another's memory space.
Access Control: Restricting which tasks can access specific resources.
Communication Security: Encrypting data transmitted between systems.
Update Mechanisms: Securely updating software in deployed systems.
Solutions like Wind River's Security Profile, Green Hills' INTEGRITY Security Services, and BlackBerry's QNX Neutrino address these challenges with comprehensive security frameworks.
Virtualizaiton and Multicore Processing
Modern embedded systems leverage multiple cores:
Partitioning: Isolating critical and non-critical components to prevent interference.
Hypervisors: Running multiple operating systems on the same hardware, combining RTOS with general-purpose OS capabilities.
Multicore Scheduling: Distributing real-time tasks across cores while maintaining timing guarantees.
Inter-Core Communications: Optimizing data sharing between tasks on different cores.
Technologies like Wind River's Helix Virtualization Platform, Green Hills' INTEGRITY Multivisor, and hypervisor-capable RTOSes address these challenges.
7. Future Trends and Emerging Technologies
AI and Machine Learning Integration
Real-time AI capabilities are becoming essential:
Inference Acceleration: Optimizing neural network execution for deterministic performance.
Mixed-Criticality Systems: Combining AI processing with traditional real-time control tasks.
Online Learning: Developing techniques for safe adaptation while maintaining real-time guarantees.
Predictive Maintenance: Using AI to anticipate system failures before they occur.
8. Conclusion
Real-Time Operating Systems remain a critical foundation for systems where timing predictability is essential. From the Mars rovers to the anti-lock brakes in your car, from life-supporting medical devices to the telecommunications infrastructure connecting our world, RTOS technologies enable the reliable operation of countless systems we depend on daily.
As embedded devices become more powerful and connected, the line between RTOS and general-purpose operating systems continues to blur. Modern RTOS now support rich networking, graphics, and storage capabilities while maintaining their core guarantee of deterministic timing. The integration of AI capabilities, increased security features, and support for multicore processing represent the next frontier in RTOS evolution.
For embedded systems developers, understanding RTOS principles and implementation methodologies remains an essential skill. The choice of RTOS—whether commercial, open-source, or custom—should be guided by application requirements, certification needs, and development ecosystem considerations.
As we advance into an era of autonomous vehicles, intelligent medical devices, and industrial IoT, RTOS will continue to evolve, providing the deterministic foundation upon which increasingly sophisticated real-time applications can be built. The fundamental guarantee of "right answer, right time, every time" remains as relevant today as when RTOS first emerged decades ago.
References
Kopetz, H. (2011). Real-Time Systems: Design Principles for Distributed Embedded Applications. Springer.
Wind River Systems (2023). VxWorks Programmer's Guide, Version 7.
Liu, J. W. S. (2000). Real-Time Systems. Prentice Hall.
Buttazzo, G. C. (2011). Hard Real-Time Computing Systems: Predictable Scheduling Algorithms and Applications. Springer.
BlackBerry QNX (2022). QNX Neutrino RTOS: System Architecture Guide.
Amazon Web Services (2023). FreeRTOS User Guide.
Laplante, P. A., & Ovaska, S. J. (2011). Real-Time Systems Design and Analysis: Tools for the Practitioner. Wiley-IEEE Press.
Green Hills Software (2022). INTEGRITY RTOS Reference Manual.
NASA/JPL (2021). Mars 2020 Perseverance Rover: Software Systems Reference.
Article Info
Engage
Table of Contents
- Abstract
- 1. Introduction
- 2. Historical Context and Evolution
- Origins of Real-Time Computing
- First Commercial RTOS
- Evolution and Standardization
- Modern RTOS Landscape
- 3. Technical Deep-Dive: RTOS Architecture
- Core Components
- Task Scheduling Algorithms
- Task Management
- Memory Management
- Inter-Process Communication
- Real-Time Clock and Timer Services
- 4. Implementation Methodologies
- RTOS Selection Criteria
- Development Process
- Optimization Techniques
- Common Pitfalls
- Case Study: VxWorks in Aerospace Applications
- 5. Real-World Applications
- Aerospace and Defense
- Automotive
- Industrial Automation
- Medical Devices
- Telecommunications
- Consumer Electronics
- 6. Challenges and Solutions
- Real-Time Performance Validation
- Safety and Certification
- Security Considerations
- Virtualizaiton and Multicore Processing
- 7. Future Trends and Emerging Technologies
- 8. Conclusion
- References