Gesture Recognition on NVIDIA Jetson Nano
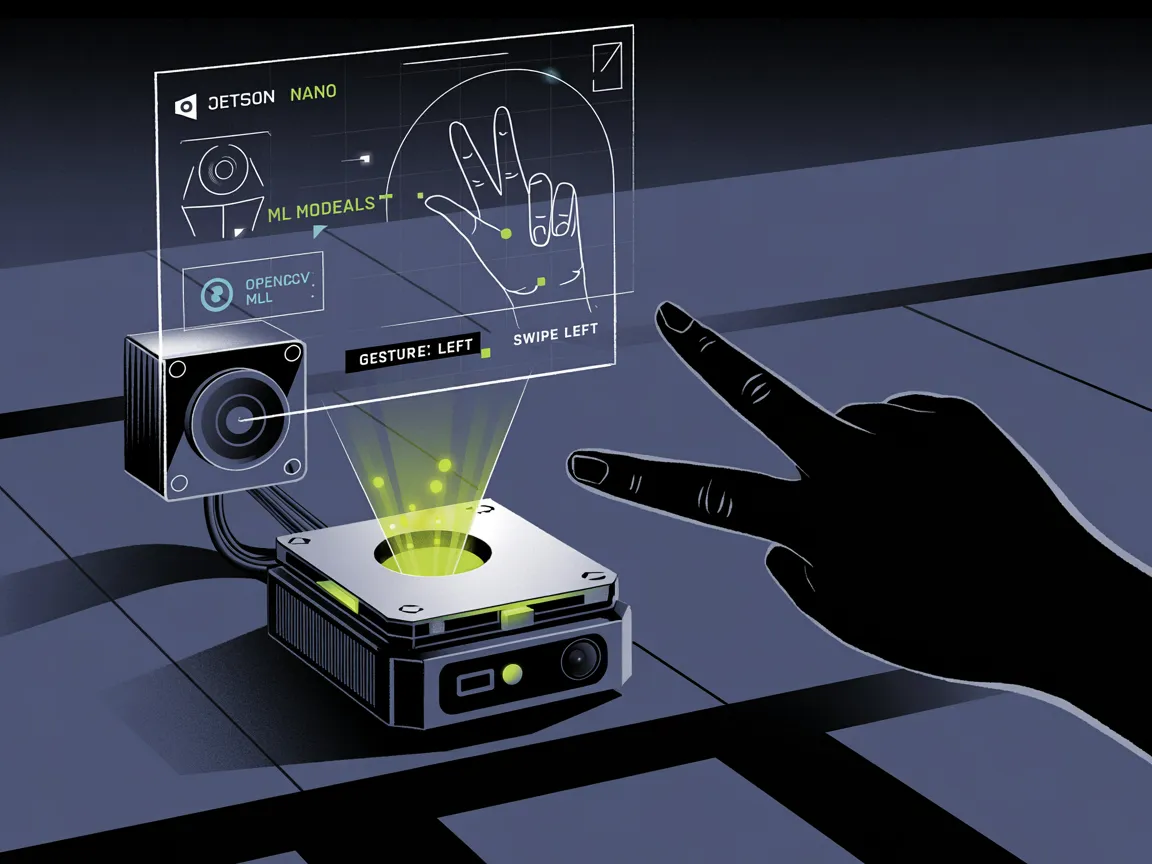
Article
Gesture recognition is a vital branch of computer vision that allows systems to interpret human gestures via mathematical algorithms. With the emergence of edge computing devices like the NVIDIA Jetson Nano, these complex technologies are now accessible to hobbyists and professionals alike. This tutorial provides a detailed guide on implementing gesture recognition using the Jetson Nano, focusing on practical implementations and addressing common challenges.
Key Takeaways:
Core Understanding: Gain insight into the foundational concepts of gesture recognition and its applications on edge devices.
Hands-On Implementation: Step-by-step guidance to set up hardware and software necessary for gesture recognition on Jetson Nano.
Machine Learning Models: Learn to train and optimize machine learning models specifically for Jetson Nano's ARM architecture.
Real-World Applications: Explore various industry use cases and case studies illustrating the deployment of gesture recognition technology.
Performance Optimization: Discover techniques to optimize model performance for resource-constrained environments.
Prerequisites
Required Hardware:
NVIDIA Jetson Nano Developer Kit (2GB or 4GB version)
USB Camera (or CSI camera modules like Raspberry Pi Camera v2)
Power Supply (5V/4A recommended for full performance)
Active cooling solution (fan or heatsink recommended for prolonged operation)
MicroSD card (32GB+ class 10 or UHS-1)
Required Software:
JetPack SDK (v4.6 or later recommended)
CUDA (version 10.2, included in JetPack)
cuDNN (version 8.x, included in JetPack)
TensorRT (for model optimization)
OpenCV (with CUDA support)
Python 3.6+
Knowledge Prerequisites:
Basic Linux command line experience
Python programming fundamentals
Basic understanding of neural networks
Introduction
Gesture recognition involves interpreting human body language into commands or actions within a digital environment. The technology is becoming increasingly important in contactless interfaces, especially in post-pandemic design paradigms. Real-world applications range from navigation in augmented reality to controlling devices in smart homes and industrial automation.
The NVIDIA Jetson Nano offers a powerful platform for developing such systems due to its:
128-core Maxwell GPU
Quad-core ARM A57 CPU
Compact form factor (69.6 mm x 45 mm)
4GB RAM (in the standard model)
Low power consumption (5-10W)
This combination makes it ideal for edge AI applications where cloud connectivity isn't reliable or latency requirements are stringent.
Implementation Guide
Step 1: Hardware Setup
1.1 Unbox and Prepare the Jetson Nano
Carefully unpack the Jetson Nano Developer Kit
Attach the heatsink/fan to the module (critical for sustained performance)
Insert a prepared microSD card with the Jetson Nano image
1.2 Connectivity and Power
Connect the Nano to a monitor via HDMI
Connect keyboard, mouse, and ethernet
Use a 5V/4A barrel jack power supply for optimal performance
Note: USB power (5V/2A) will work but may trigger throttling under heavy loads
Step 2: Software Configuration
2.1 Install JetPack SDK
Download the latest JetPack SDK image from NVIDIA's developer website
Flash the image to your microSD card using Etcher or similar software
Boot the Jetson Nano and complete the initial setup
2.2 System Updates and Dependencies
# Update the system packages
sudo apt-get update
sudo apt-get upgrade
# Install development tools
sudo apt-get install -y build-essential cmake pkg-config
# Install OpenCV dependencies
sudo apt-get install -y \
libavcodec-dev libavformat-dev libswscale-dev \
libgstreamer1.0-dev libgstreamer-plugins-base1.0-dev \
libgtk-3-dev libpng-dev libjpeg-dev
# Install Python dependencies
sudo apt-get install -y python3-dev python3-pip
sudo pip3 install -U numpy matplotlib
# Install TensorFlow for Jetson (optimized version)
sudo pip3 install --extra-index-url https://developer.download.nvidia.com/compute/redist/jp/v46 tensorflow==2.5.0+nv21.5
2.3 Install and Build OpenCV with CUDA Support
For optimal performance, building OpenCV with CUDA support is recommended. This is a time-consuming but worthwhile process for gesture recognition applications:
# Clone OpenCV and OpenCV contrib repositories
git clone https://github.com/opencv/opencv.git
git clone https://github.com/opencv/opencv_contrib.git
# Set up the build directory
cd opencv
mkdir build
cd build
# Configure the build with CUDA support
cmake -D CMAKE_BUILD_TYPE=RELEASE \
-D CMAKE_INSTALL_PREFIX=/usr/local \
-D WITH_CUDA=ON \
-D CUDA_ARCH_BIN="5.3" \
-D CUDA_ARCH_PTX="" \
-D WITH_CUBLAS=ON \
-D ENABLE_FAST_MATH=ON \
-D CUDA_FAST_MATH=ON \
-D ENABLE_NEON=ON \
-D WITH_GSTREAMER=ON \
-D WITH_LIBV4L=ON \
-D BUILD_opencv_python3=ON \
-D BUILD_opencv_python2=OFF \
-D BUILD_opencv_java=OFF \
-D WITH_QT=OFF \
-D WITH_GTK=ON \
-D BUILD_TESTS=OFF \
-D BUILD_PERF_TESTS=OFF \
-D BUILD_EXAMPLES=OFF \
-D OPENCV_EXTRA_MODULES_PATH=../../opencv_contrib/modules \
..
# Compile (adjust -j based on available cores, typically -j4 for Jetson Nano)
make -j4
# Install
sudo make install
sudo ldconfig
Step 3: Gesture Recognition Approaches
There are three main approaches to implementing gesture recognition on the Jetson Nano:
3.1 Pre-trained Models (Fastest to Implement)
For rapid prototyping, you can leverage pre-trained models compatible with Jetson Nano:
import cv2
import numpy as np
import tensorflow as tf
# Load a pre-trained hand detection model
# TensorFlow Lite is often a good choice for Jetson Nano
interpreter = tf.lite.Interpreter(model_path="hand_landmark_lite.tflite")
interpreter.allocate_tensors()
# Get input and output tensors
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
# Set up camera
camera = cv2.VideoCapture(0)
camera.set(cv2.CAP_PROP_FRAME_WIDTH, 640)
camera.set(cv2.CAP_PROP_FRAME_HEIGHT, 480)
while True:
ret, frame = camera.read()
if not ret:
break
# Preprocess image for the model
img = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
img = cv2.resize(img, (input_details[0]['shape'][1], input_details[0]['shape'][2]))
img = np.expand_dims(img, axis=0)
img = (img / 127.5) - 1 # Normalize to [-1, 1]
# Run inference
interpreter.set_tensor(input_details[0]['index'], img.astype(np.float32))
interpreter.invoke()
# Get results
landmarks = interpreter.get_tensor(output_details[0]['index'])
# Process landmarks and visualize
# [Implementation depends on model output format]
# Display result
cv2.imshow('Gesture Recognition', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
camera.release()
cv2.destroyAllWindows()
3.2 Custom Model Training (Most Flexible)
For applications requiring custom gestures, training your own model is recommended:
Data Collection:
import cv2
import os
import time
# Create directory structure
gestures = ["thumbs_up", "peace", "open_palm", "fist", "pointing"]
for gesture in gestures:
os.makedirs(f"dataset/{gesture}", exist_ok=True)
# Set up camera
camera = cv2.VideoCapture(0)
camera.set(cv2.CAP_PROP_FRAME_WIDTH, 640)
camera.set(cv2.CAP_PROP_FRAME_HEIGHT, 480)
for gesture in gestures:
print(f"Prepare to capture {gesture}")
time.sleep(5) # Give user time to prepare
print(f"Capturing {gesture} - 3 seconds")
# Capture 30 frames (approx. 1 second) for each gesture
for i in range(30):
ret, frame = camera.read()
if ret:
filename = f"dataset/{gesture}/{time.time()}.jpg"
cv2.imwrite(filename, frame)
cv2.imshow('Capture', frame)
cv2.waitKey(100) # Short delay between captures
camera.release()
cv2.destroyAllWindows()
Model Training (typically done on a more powerful machine and then transferred to Jetson):
import tensorflow as tf
from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.applications import MobileNetV2
from tensorflow.keras.layers import Dense, GlobalAveragePooling2D
from tensorflow.keras.models import Model
# Data augmentation
datagen = ImageDataGenerator(
rescale=1./255,
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
validation_split=0.2
)
# Load training data
train_generator = datagen.flow_from_directory(
'dataset',
target_size=(224, 224),
batch_size=32,
class_mode='categorical',
subset='training'
)
# Load validation data
validation_generator = datagen.flow_from_directory(
'dataset',
target_size=(224, 224),
batch_size=32,
class_mode='categorical',
subset='validation'
)
# Create a base model from MobileNetV2 (efficient for edge devices)
base_model = MobileNetV2(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# Freeze the base model
base_model.trainable = False
# Add custom classification head
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(128, activation='relu')(x)
predictions = Dense(len(gestures), activation='softmax')(x)
# Create the final model
model = Model(inputs=base_model.input, outputs=predictions)
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Train the model
history = model.fit(
train_generator,
steps_per_epoch=train_generator.samples // train_generator.batch_size,
epochs=10,
validation_data=validation_generator,
validation_steps=validation_generator.samples // validation_generator.batch_size
)
# Save the model
model.save('gesture_recognition_model.h5')
# Convert to TensorFlow Lite for better performance on Jetson
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
with open('gesture_recognition_model.tflite', 'wb') as f:
f.write(tflite_model)
Model Optimization for Jetson Nano:
# Convert TensorFlow model to TensorRT
/usr/src/tensorrt/bin/trtexec --onnx=gesture_model.onnx --saveEngine=gesture_model.trt
3.3 Algorithmic Approach (Lowest Resource Usage)
For simple gestures, traditional computer vision techniques may be sufficient:
import cv2
import numpy as np
def detect_hand(frame):
# Convert to HSV color space
hsv = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
# Define range for skin color in HSV
lower_skin = np.array([0, 20, 70], dtype=np.uint8)
upper_skin = np.array([20, 255, 255], dtype=np.uint8)
# Create a binary mask
mask = cv2.inRange(hsv, lower_skin, upper_skin)
# Apply morphological operations to reduce noise
kernel = np.ones((5,5), np.uint8)
mask = cv2.dilate(mask, kernel, iterations=2)
mask = cv2.erode(mask, kernel, iterations=2)
# Find contours
contours, _ = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
if contours:
# Find largest contour (assumed to be the hand)
max_contour = max(contours, key=cv2.contourArea)
# Calculate convex hull and defects
hull = cv2.convexHull(max_contour, returnPoints=False)
defects = cv2.convexityDefects(max_contour, hull)
# Count fingers
finger_count = 0
if defects is not None:
for i in range(defects.shape[0]):
s, e, f, d = defects[i, 0]
start = tuple(max_contour[s][0])
end = tuple(max_contour[e][0])
far = tuple(max_contour[f][0])
# Calculate angle between fingers
a = np.sqrt((end[0] - start[0])**2 + (end[1] - start[1])**2)
b = np.sqrt((far[0] - start[0])**2 + (far[1] - start[1])**2)
c = np.sqrt((end[0] - far[0])**2 + (end[1] - far[1])**2)
angle = np.arccos((b**2 + c**2 - a**2) / (2*b*c))
# If angle is less than 90 degrees, treat as finger
if angle < np.pi/2:
finger_count += 1
# Draw contour and convex hull
cv2.drawContours(frame, [max_contour], -1, (0, 255, 0), 2)
return frame, finger_count
return frame, 0
# Set up camera
camera = cv2.VideoCapture(0)
camera.set(cv2.CAP_PROP_FRAME_WIDTH, 640)
camera.set(cv2.CAP_PROP_FRAME_HEIGHT, 480)
while True:
ret, frame = camera.read()
if not ret:
break
# Process frame
processed_frame, fingers = detect_hand(frame)
# Display finger count
cv2.putText(processed_frame, f"Fingers: {fingers}", (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
# Display result
cv2.imshow('Hand Gesture Recognition', processed_frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
camera.release()
cv2.destroyAllWindows()
Step 4: Performance Optimization
Optimizing for the Jetson Nano's resource constraints is critical:
4.1 Model Quantization
Convert floating-point weights to 8-bit integers to reduce memory usage and increase inference speed:
# During TFLite conversion
converter = tf.lite.TFLiteConverter.from_keras_model(model)
converter.optimizations = [tf.lite.Optimize.DEFAULT]
quantized_model = converter.convert()
4.2 GPU Acceleration
Ensure your model uses GPU acceleration through CUDA:
# Check if CUDA is available
print(f"CUDA available: {tf.test.is_gpu_available()}")
print(f"GPU devices: {tf.config.list_physical_devices('GPU')}")
# Set memory growth
gpus = tf.config.experimental.list_physical_devices('GPU')
if gpus:
try:
for gpu in gpus:
tf.config.experimental.set_memory_growth(gpu, True)
except RuntimeError as e:
print(e)
4.3 Frame Processing Optimization
Reduce input resolution and processing frequency:
# Reduce resolution
camera.set(cv2.CAP_PROP_FRAME_WIDTH, 320)
camera.set(cv2.CAP_PROP_FRAME_HEIGHT, 240)
# Process every other frame
process_frame = True
while True:
ret, frame = camera.read()
if process_frame:
# Process frame
process_frame = not process_frame
Common Challenges
1. Limited Processing Power
Solution: Use model quantization, reduce input resolution, and optimize inference pipelines. Consider using TensorRT for model optimization.
2. Power Consumption
Solution: Implement dynamic frequency scaling based on load, reduce unnecessary processing, and use efficient algorithms.
3. Thermal Management
Solution: Ensure adequate cooling with a heatsink and fan. Monitor temperature and throttle processing if necessary:
def get_jetson_temperature():
with open("/sys/devices/virtual/thermal/thermal_zone0/temp", "r") as f:
temp = int(f.read().strip()) / 1000
return temp
# In main loop
temp = get_jetson_temperature()
if temp > 80: # 80°C threshold
# Reduce processing load
4. Lighting Variability
Solution: Implement adaptive thresholding, use HSV color space for skin detection, and employ data augmentation during training.
Realistic Benchmarking
Performance varies significantly based on model complexity and implementation approach:
Model TypeResolutionFPSPower ConsumptionAccuracyTensorFlow Lite (Quant.)224x22412-15~7W92%TensorRT Optimized224x22418-22~8W92%OpenCV Algorithmic320x24025-30~5W85%Full TensorFlow (Float)224x2245-8~10W94%
Note: These figures represent typical performance on a Jetson Nano 4GB with active cooling. Your results may vary based on specific implementation details.
Industry Applications
1. Healthcare
Contactless Interfaces: Critical in operating rooms and sterile environments
Rehabilitation: Motion tracking for physical therapy
Case Study: A medical imaging company implemented gesture control for surgeons to manipulate 3D scans during procedures without breaking sterility
2. Automotive
Driver Monitoring: Detect driver fatigue or distraction
Infotainment Control: Reduce driver distraction by enabling gesture-based control
Case Study: A major automotive supplier integrated gesture recognition on Jetson Nano for an aftermarket driver monitoring system
3. Smart Home and IoT
Device Control: Gesture-based interaction with home appliances
Accessibility: Enhanced interfaces for users with mobility impairments
Case Study: A startup developed a Jetson Nano-powered hub that enables gesture control of existing smart home devices
Conclusion
Gesture recognition on the NVIDIA Jetson Nano demonstrates the capabilities of edge AI in real-world applications. The platform offers a compelling balance of processing power and energy efficiency, making it suitable for deployment in diverse environments.
Key considerations for successful implementation include:
Appropriate Model Selection: Choose the right approach based on your specific requirements and constraints
Optimization: Tailor your solution to the Jetson Nano's capabilities
Environmental Factors: Consider lighting, background, and user variability in your design
As edge AI continues to evolve, we can expect even more powerful and efficient implementations of gesture recognition technology, enabling more natural and intuitive human-computer interaction across diverse applications.
References
NVIDIA. (2023). Jetson Nano Developer Kit Documentation. https://developer.nvidia.com/embedded/learn/get-started-jetson-nano-devkit
Géron, A. (2022). Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow. O'Reilly Media.
Bradski, G. & Kaehler, A. (2022). Learning OpenCV 5: Computer Vision with Python. O'Reilly Media.
Sandler, M., Howard, A., Zhu, M., Zhmoginov, A., & Chen, L. C. (2018). MobileNetV2: Inverted Residuals and Linear Bottlenecks. Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition, 4510-4520.
Zhang, F., Bazarevsky, V., Vakunov, A., Tkachenka, A., Sung, G., Chang, C. L., & Grundmann, M. (2020). MediaPipe Hands: On-device Real-time Hand Tracking. arXiv preprint arXiv:2006.10214.
Article Info
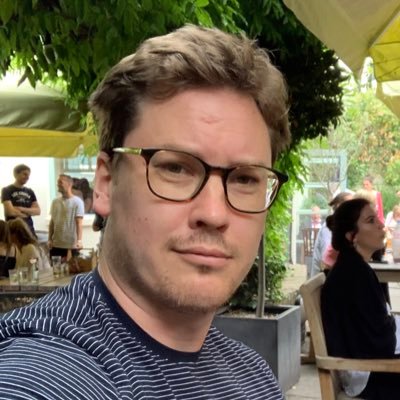
Ben
Published March 31, 2025
Engage
Table of Contents
- Key Takeaways:
- Prerequisites
- Required Hardware:
- Required Software:
- Knowledge Prerequisites:
- Introduction
- Implementation Guide
- Step 1: Hardware Setup
- Step 2: Software Configuration
- 2.1 Install JetPack SDK
- 2.2 System Updates and Dependencies
- 2.3 Install and Build OpenCV with CUDA Support
- Step 3: Gesture Recognition Approaches
- 3.1 Pre-trained Models (Fastest to Implement)
- 3.2 Custom Model Training (Most Flexible)
- 3.3 Algorithmic Approach (Lowest Resource Usage)
- Step 4: Performance Optimization
- Common Challenges
- 1. Limited Processing Power
- 2. Power Consumption
- 3. Thermal Management
- 4. Lighting Variability
- Realistic Benchmarking
- Industry Applications
- 1. Healthcare
- 2. Automotive
- 3. Smart Home and IoT
- Conclusion
- References