Federated Learning: Decentralized AI Without Sacrificing Data Privacy
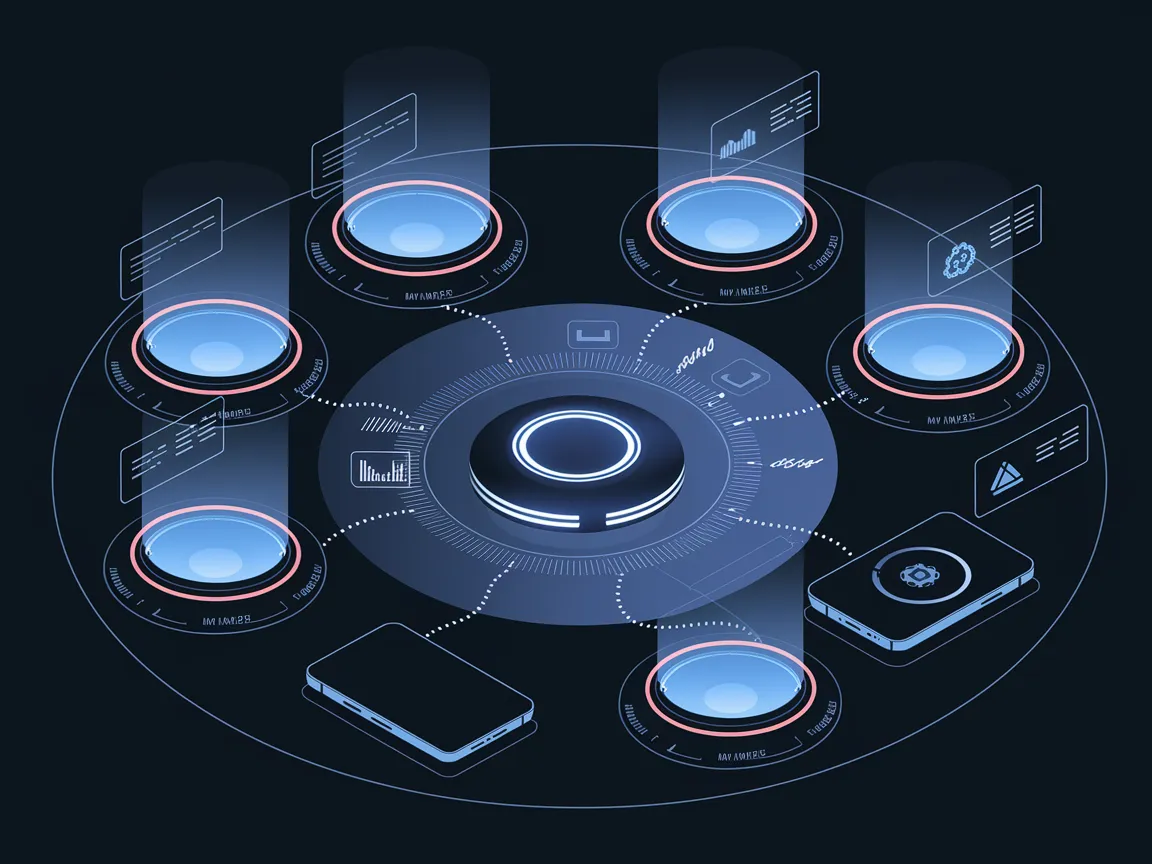
Article
Federated Learning (FL) is revolutionizing how we train AI models in an era dominated by data privacy concerns. Instead of pooling sensitive user data into a central location, FL enables collaborative model training directly on distributed devices like smartphones or hospital servers. This technical guide unpacks the mechanics of FL, demonstrating its power to build robust models while upholding strict data locality. We'll explore the fundamental workflow, provide hands-on implementation steps using TensorFlow Federated, tackle common challenges like data heterogeneity, and showcase FL's transformative potential in sectors like healthcare and autonomous systems. Key takeaways include:
Privacy by Design: FL trains models collaboratively across decentralized data sources, keeping raw data localized and secure.
Core Mechanisms: Understand the iterative process of local training, secure aggregation, and global model updates central to FL.
Practical Implementation: Get started with TensorFlow Federated to build and train a simple federated model.
Navigating Challenges: Address critical issues like non-IID data, communication bottlenecks, and security vulnerabilities with practical strategies.
Real-World Impact & Future: See FL in action (e.g., mobile keyboards, medical research) and explore its trajectory in the evolving AI landscape.
Prerequisites
Before diving in, ensure you have the following setup:
Programming Language: Python 3.8+ (familiarity assumed)
Core ML Libraries:
TensorFlow 2.x (for the model definition and local training logic)
NumPy (for numerical operations)
Federated Learning Framework:
TensorFlow Federated (TFF) - We'll use this for orchestrating the FL process. (Note: Other frameworks like PySyft for PyTorch exist, but concepts are similar.)
Development Environment: Jupyter Notebook, Google Colab, or a Python IDE (PyCharm, VSCode). A virtual environment (venv or conda) is highly recommended.
Setup Instructions:
Install/Update Python: python.org
Create and activate a virtual environment (optional but recommended).
Install libraries via pip:
pip install --upgrade pip pip install tensorflow numpy tensorflow-federated # Consider installing specific versions if needed for compatibility
Introduction: The Data Privacy Paradox in AI
Modern AI thrives on data. Yet, collecting vast amounts of user data centrally poses significant privacy risks and logistical hurdles, especially with regulations like GDPR and CCPA. How can we leverage diverse, real-world data for powerful AI without compromising user privacy?
Enter Federated Learning (FL). Proposed by Google researchers in 2016/2017, FL offers an elegant solution: bring the model to the data, not the data to the model.
Imagine training a predictive keyboard model. Instead of uploading sensitive user typing data to a central server, FL allows the model to be trained directly on thousands or millions of individual smartphones. Each phone improves a local version of the model based on its user's typing patterns. Only these model updates (e.g., weight changes), not the raw text data, are sent back (often encrypted and aggregated) to a central server to improve a shared global model.
This decentralized approach is transformative:
Privacy Preservation: Raw, potentially sensitive data never leaves the user's device or local environment (e.g., a hospital's server).
Reduced Communication Costs: Sending small model updates can be more efficient than transmitting large raw datasets.
Access to Diverse Data: Enables training on heterogeneous, real-world data distributions that are difficult or impossible to centralize.
FL is already powering features like Google's Gboard predictions and enabling collaborative medical research across hospitals without sharing patient records.
Core Concepts: How Federated Learning Works
The most common FL approach, Federated Averaging (FedAvg), follows an iterative process orchestrated by a central server:
Initialization: The server starts with an initial global model.
Distribution: The server sends the current global model to a selected subset of participating clients (e.g., devices, organizations).
Local Training: Each selected client trains the received model on its local data for a few epochs. This generates a personalized model update reflecting its local data patterns.
Update Transmission: Clients send their computed model updates (e.g., weight differentials or updated weights) back to the server. Crucially, the raw local data is notsent. Techniques like Secure Aggregation can be used here to ensure the server only sees the combined result, not individual updates.
Aggregation: The server aggregates the updates from multiple clients (e.g., by averaging them, weighted by the amount of data each client used).
Global Model Update: The server uses the aggregated update to refine the global model.
Iteration: Repeat steps 2-6 for multiple communication rounds until the global model converges or reaches desired performance.
Implementation Guide with TensorFlow Federated (TFF)
Let's walk through building a basic FL system using TFF. We'll simulate a scenario with multiple clients, each having its own local data.
(Note: This example uses a simplified setup for clarity. Real-world FL involves handling device availability, asynchronous updates, and more complex data pipelines.)
Step 1: Environment Setup
Ensure you've completed the setup mentioned in the Prerequisites section.
Step 2: Prepare Federated Data
In a real FL scenario, data resides on distributed clients. TFF provides tools to simulate this. Let's imagine we have a dataset (like MNIST digits) partitioned among several clients.
import collections
import numpy as np
import tensorflow as tf
import tensorflow_federated as tff
# Load a sample dataset (e.g., MNIST) and preprocess it
(mnist_train, mnist_test), _ = tf.keras.datasets.mnist.load_data()
# Flatten, normalize, and structure the data
def preprocess(dataset):
def batch_format_fn(element):
# Flatten image and normalize pixel values
return (tf.reshape(element['pixels'], [-1, 784]) / 255.0,
tf.reshape(element['label'], [-1, 1]))
return dataset.map(lambda x: {'pixels': x[0], 'label': x[1]}) \
.shuffle(1000).batch(20).map(batch_format_fn)
# Simulate distributing data across 10 clients (non-IID is common in FL)
NUM_CLIENTS = 10
NUM_EPOCHS = 5 # Local epochs per client round
BATCH_SIZE = 20
SHUFFLE_BUFFER = 100
# Create a list of datasets, one per client
# In a real scenario, data is already at the clients. Here we simulate partitioning.
source = tf.data.Dataset.from_tensor_slices(
collections.OrderedDict([('pixels', mnist_train[0]), ('label', mnist_train[1])])
)
# Simple partitioning (real FL data is often naturally partitioned and non-IID)
federated_train_data = [
preprocess(source.skip(i * (len(mnist_train[0]) // NUM_CLIENTS))
.take(len(mnist_train[0]) // NUM_CLIENTS))
for i in range(NUM_CLIENTS)
]
print(f"Created {len(federated_train_data)} simulated client datasets.")
# Example: Access data for the first client
sample_batch = tf.nest.map_structure(lambda x: x.numpy(), next(iter(federated_train_data[0])))
print("Sample batch structure:", sample_batch)
Step 3: Define the Machine Learning Model
Define a standard Keras model. TFF will wrap this for use in the federated setting.
def create_keras_model():
# Simple MLP for MNIST
return tf.keras.models.Sequential([
tf.keras.layers.InputLayer(input_shape=(784,)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax') # 10 classes for MNIST digits
])
# Verify model structure
keras_model = create_keras_model()
keras_model.summary()
Step 4: Wrap the Model and Define the FL Process
TFF requires the model logic to be wrapped in tff.learning.Model. It also provides high-level builders like build_federated_averaging_process for common FL algorithms.
# Wrap the Keras model for TFF compatibility
# Need to define dummy batch to determine input/output specs
def model_fn():
keras_model = create_keras_model()
return tff.learning.from_keras_model(
keras_model,
# Define input spec based on preprocessed data
input_spec=federated_train_data[0].element_spec,
loss=tf.keras.losses.SparseCategoricalCrossentropy(),
metrics=[tf.keras.metrics.SparseCategoricalAccuracy()])
# Build the Federated Averaging process
# This encapsulates the orchestration logic (distribution, client training, aggregation)
federated_averaging_process = tff.learning.build_federated_averaging_process(
model_fn,
# Optimizers for server (updating global model) and client (local training)
server_optimizer_fn=lambda: tf.keras.optimizers.Adam(learning_rate=1.0), # Server learning rate often 1.0 for FedAvg
client_optimizer_fn=lambda: tf.keras.optimizers.SGD(learning_rate=0.02)
)
print("Federated Averaging process created.")
Step 5: Train the Federated Model
Now, execute the federated training loop.
# Initialize the FL process (creates the initial server state/model)
state = federated_averaging_process.initialize()
NUM_ROUNDS = 10 # Number of communication rounds
print("Starting Federated Training...")
for round_num in range(1, NUM_ROUNDS + 1):
try:
# Select a subset of clients for this round (here, we use all clients for simplicity)
# In practice, sample clients: sampled_clients_data = np.random.choice(federated_train_data, size=..., replace=False)
sampled_clients_data = federated_train_data
# Run one round of Federated Averaging
# state: contains the global model weights
# sampled_clients_data: list of tf.data.Datasets for selected clients
state, metrics = federated_averaging_process.next(state, sampled_clients_data)
# Print metrics for the round
# 'metrics' contains aggregated results like loss and accuracy
print(f"Round {round_num}: Metrics = {metrics['train']}")
except Exception as e:
print(f"Error during training round {round_num}: {e}")
# Add more robust error handling/logging as needed
break # Stop training on error in this simple example
print("Federated Training finished.")
# The final global model weights are in 'state.model'
# You can extract and evaluate this model on a centralized test set if available.
Common Challenges and Mitigation Strategies
FL isn't without its complexities:
Statistical Heterogeneity (Non-IID Data):
Issue: Client data distributions vary significantly (e.g., different users type different words, hospitals see different patient demographics). This can slow convergence or lead to models that perform poorly for some clients.
Solutions:
Personalization: Techniques like fine-tuning the global model on local data (e.g., using meta-learning approaches like Reptile) or clustering clients (e.g., Federated Multi-Task Learning).
Robust Aggregation: Algorithms beyond simple FedAvg that are less sensitive to outliers or skewed updates (e.g., FedProx, SCAFFOLD).
Systems Heterogeneity:
Issue: Clients have varying hardware (CPU, memory), network connectivity (bandwidth, latency), and availability (devices going offline).
Solutions:
Asynchronous FL: Allow clients to join training and send updates when ready, rather than waiting for fixed rounds.
Device Sampling: Strategically select clients based on readiness or capability.
Adaptive Algorithms: Adjust local computation or communication frequency based on device status.
Communication Bottlenecks:
Issue: Frequent communication of large model updates can be costly, especially for mobile devices or over slow networks.
Solutions:
Model Compression: Techniques like quantization (using lower precision numbers), sparsification (sending only significant weights), or structured updates (sending low-rank approximations).
Less Frequent Updates: Perform more local computation (epochs) per communication round.
Privacy and Security Concerns:
Issue: While raw data stays local, model updates can potentially leak information about the underlying data through sophisticated attacks (e.g., model inversion, membership inference).
Solutions:
Secure Aggregation (SecAgg): Use cryptographic techniques (like multi-party computation - MPC) so the server only sees the sum of updates, not individual client contributions.
Differential Privacy (DP): Inject carefully calibrated noise into client updates before aggregation, providing mathematical guarantees about individual privacy, albeit often at the cost of some model accuracy.
Advanced Techniques in Federated Learning
Beyond basic FedAvg, the field is rapidly evolving:
Personalized Federated Learning (PFL): Aims to provide models tailored to individual clients or groups, rather than a single global model. Methods include:
Fine-tuning: Train a global model via FL, then fine-tune it on each client's local data.
Meta-Learning: Learn an initial model that can be rapidly adapted to new clients/tasks (e.g., FedMeta, Reptile).
Clustering: Group clients with similar data and train separate models for each cluster.
Federated Transfer Learning: Leverage knowledge from pre-trained models (on public data) within the FL framework to speed up training or improve performance, especially with limited local data.
Vertical Federated Learning (VFL): Handles scenarios where different entities hold different features for the same set of samples (e.g., a bank and an e-commerce company have different data about the same customers). Requires careful coordination and encryption during feature alignment and model training.
Cross-Silo vs. Cross-Device FL:
Cross-Silo: Fewer, more reliable clients (e.g., organizations, hospitals) with larger datasets. Typically involves fewer communication rounds but more computation per client.
Cross-Device: Massive numbers of less reliable clients (e.g., mobile phones) with smaller datasets and limited resources. Requires fault tolerance and efficient communication.
Benchmarking and Evaluation
Evaluating FL models requires careful consideration:
Methodology
Metrics: Standard ML metrics (accuracy, precision, recall, F1-score, AUC) are used, but often need to be evaluated both for the global model (on a held-out test set) and potentially for personalized models on local client test data.
Fairness: Assess performance disparities across different clients or subgroups to ensure the model isn't unfairly biased.
Communication Cost: Track the total data transmitted during training.
Convergence Speed: Measure the number of communication rounds needed to reach target performance.
Simulation vs. Real-World: Benchmarking often starts in simulation (like our TFF example), but validation in real, heterogeneous environments is crucial.
Illustrative Results (Hypothetical Example)
Scenario | Avg. Global Accuracy | Communication Cost (Rel.) | Notes |
Centralized Training | 95% | N/A (Data Centralized) | Upper bound (if data could be pooled) |
Basic FedAvg (IID Data) | 93% | Medium | Ideal conditions, rarely occur in practice |
Basic FedAvg (Non-IID) | 88% | Medium | Performance drop due to heterogeneity |
FedAvg + DP | 85% | Medium | Privacy gain, potential accuracy trade-off |
Personalized FL (Non-IID) | 91% (Avg. Local Acc) | High | Better local performance, more complex |
FedAvg + Compression | 87% | Low | Reduced communication, slight accuracy dip |
Interpretation
Centralized training often sets the performance ceiling, assuming data could be pooled (which FL avoids).
FL performance is heavily influenced by data heterogeneity (Non-IID). Basic FedAvg can struggle here.
Advanced techniques like PFL can recover performance on Non-IID data but may increase complexity or communication.
Privacy-enhancing techniques like DP introduce a trade-off between privacy guarantees and model utility.
Real-World Industry Applications
FL is moving from research labs into production systems:
Mobile Devices:
Google Gboard: Improves next-word prediction and auto-correction based on local typing patterns without sending text to Google servers.
Apple: Uses FL for features like "Hey Siri" voice recognition personalization and QuickType suggestions.
Healthcare:
Drug Discovery: Train models across pharmaceutical companies or research labs without sharing proprietary chemical data.
Medical Imaging: Develop diagnostic models (e.g., for tumor detection) by training on patient scans from multiple hospitals without moving sensitive patient data. Projects like NVIDIA FLARE and Owkin facilitate this.
Wearable Devices: Analyze health data (e.g., ECG, activity levels) from wearables for early disease detection or mental health monitoring while preserving user privacy.
Finance:
Fraud Detection: Banks can collaboratively train fraud detection models without sharing confidential customer transaction data.
Credit Risk Assessment: Improve models by leveraging insights from different financial institutions under privacy constraints.
Autonomous Vehicles:
Predictive Maintenance: Analyze sensor data from fleets of vehicles to predict part failures without uploading raw sensor streams.
Collaborative Perception/Mapping: Improve environment understanding or map accuracy by aggregating processed sensor information from multiple vehicles while preserving location privacy.
The Future of Federated Learning
FL is a cornerstone of privacy-preserving AI and trustworthy ML. Future directions include:
Improved Efficiency: Developing more communication-efficient algorithms and hardware acceleration for FL on edge devices.
Enhanced Robustness & Fairness: Better handling of non-IID data, adversarial attacks specific to FL, and ensuring equitable performance across diverse clients.
Hybrid Approaches: Combining FL with other privacy technologies like Differential Privacy, Homomorphic Encryption, and Secure Multi-Party Computation for layered security.
Democratization: Easier-to-use frameworks and platforms to make FL accessible to more developers and organizations.
New Applications: Expanding FL into areas like industrial IoT, smart cities, and personalized recommendations.
Conclusion
Federated Learning represents a fundamental shift in building AI systems, moving away from data centralization towards collaborative, privacy-preserving intelligence. While challenges remain, the ongoing advancements in algorithms, frameworks like TensorFlow Federated, and a growing ecosystem make FL an increasingly viable and essential tool for developers and organizations. By allowing models to learn from diverse, decentralized data without compromising privacy, FL is not just a technical solution but a critical enabler for ethical and trustworthy AI in the years to come. Experimenting with FL today can provide a significant edge in building next-generation, privacy-aware applications.
References
Kairouz, P. et al. "Advances and Open Problems in Federated Learning". Foundations and Trends in Machine Learning, 2021. (Updated reference year might be available)
McMahan, H.B. et al. "Communication-efficient learning of deep networks from decentralized data". AISTATS, 2017.
Li, T., et al. "Federated Learning: Challenges, Methods, and Future Directions". IEEE Signal Processing Magazine, 2020. (Alternative comprehensive survey)
Rieke, N. et al. "The future of digital health with federated learning". NPJ Digital Medicine, 2020.
Bonawitz, K. et al. "Towards Federated Learning at Scale: System Design". SysML, 2019. (Focuses on system aspects)
TensorFlow Federated Documentation: https://www.tensorflow.org/federated
PySyft (PyTorch FL Framework): https://github.com/OpenMined/PySyft
Related Articles
Article Info
Engage
Table of Contents
- Prerequisites
- Introduction: The Data Privacy Paradox in AI
- Core Concepts: How Federated Learning Works
- Implementation Guide with TensorFlow Federated (TFF)
- Step 1: Environment Setup
- Step 2: Prepare Federated Data
- Step 3: Define the Machine Learning Model
- Step 4: Wrap the Model and Define the FL Process
- Step 5: Train the Federated Model
- Common Challenges and Mitigation Strategies
- Advanced Techniques in Federated Learning
- Benchmarking and Evaluation
- Real-World Industry Applications
- The Future of Federated Learning
- Conclusion
- References