Stereo Vision Hacking: A Comprehensive Guide to Computer Vision Projects using Jetson and Multiple Cameras
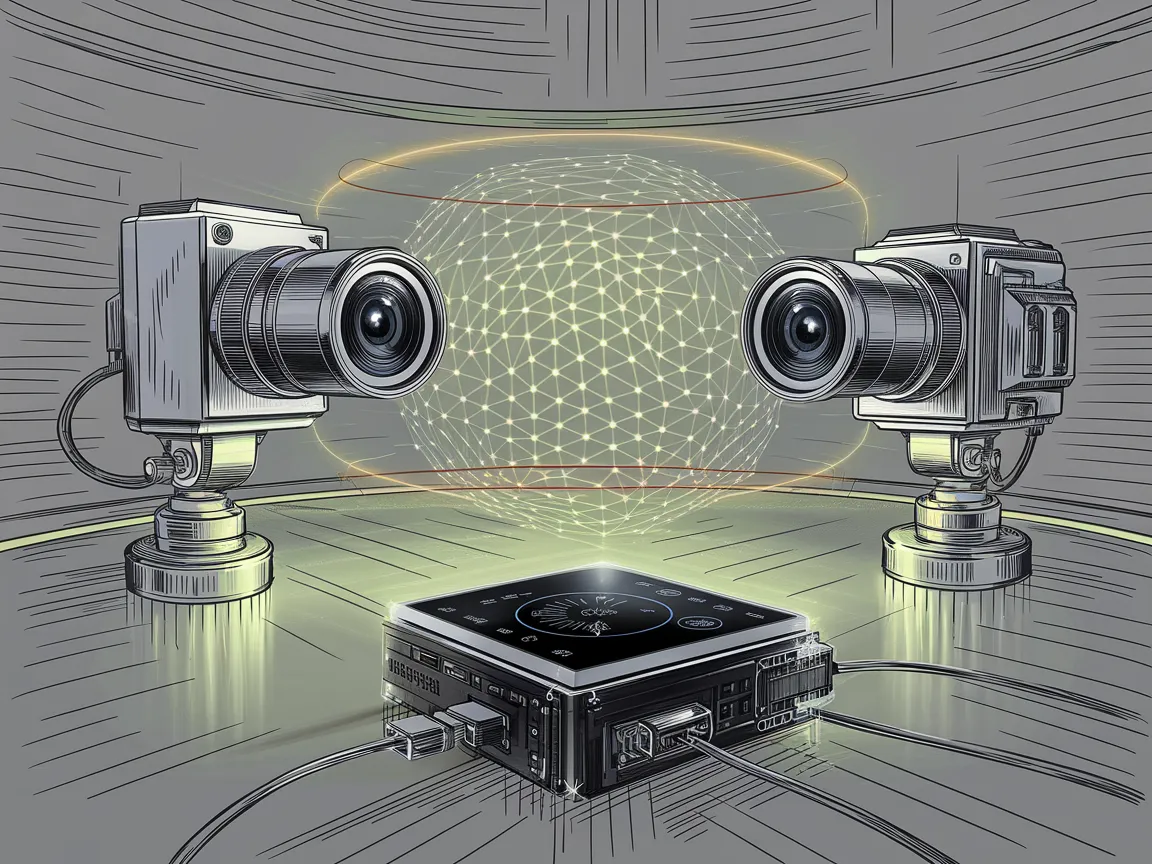
Article
Introduction: Seeing the World in 3D with Jetson
Humans perceive depth effortlessly thanks to our two eyes – a biological stereo vision system. In the world of machines, replicating this ability unlocks incredible potential. Stereo vision, using two or more cameras, allows computers to calculate depth information, transforming flat images into rich 3D representations of the environment. This capability is revolutionizing fields like robotics, autonomous navigation, augmented reality, and industrial automation.
Imagine a robot navigating a cluttered warehouse, an autonomous drone mapping terrain, or a self-driving car gauging the distance to pedestrians. These complex tasks rely heavily on understanding the 3D structure of the world, and stereo vision provides a powerful, passive way (unlike Lidar which emits signals) to achieve this.
NVIDIA Jetson platforms, with their powerful GPUs optimized for AI and parallel processing, combined with multiple camera interface (CSI) ports, are ideal "hacking" platforms for building real-time stereo vision systems at the edge.
This tutorial is your comprehensive guide to getting started with stereo vision on Jetson. We'll cover:
Core Concepts: Understanding disparity, epipolar geometry, and depth calculation.
Hardware Setup: Connecting your cameras to the Jetson.
Software Environment: Installing necessary libraries like OpenCV and jetmulticam.
Camera Calibration: The crucial step for accurate results.
Multi-Camera Capture: Efficiently grabbing frames using jetmulticam.
Image Rectification & Disparity: Aligning images and calculating the disparity map.
Depth Mapping: Converting disparity to real-world depth.
Optimization: Speeding things up with Jetson's capabilities.
Real-world Examples: Seeing stereo vision in action.
Ready to give your Jetson the power of 3D sight? Let's dive in!
Understanding the Magic: Core Stereo Vision Concepts
Before we start wiring and coding, let's grasp the fundamentals:
The Setup: Two cameras are mounted a fixed, known distance apart (the baseline), looking roughly in the same direction.
Disparity: An object appears in slightly different positions in the left and right camera images. This difference in horizontal position is called disparity. Closer objects have larger disparity; farther objects have smaller disparity.
Epipolar Geometry: This is the geometry of stereo vision. For any point in one image, its corresponding point in the other image must lie on a specific line called the epipolar line. This constraint significantly speeds up the search for matching points between the two images.
Rectification: To simplify finding correspondences, we mathematically "warp" the images so that the epipolar lines become horizontal scanlines. This means a point on row y in the left image will have its corresponding point also on row y in the right image.
Triangulation: Once we find corresponding points in the rectified images and calculate their disparity, we can use the known camera parameters (focal length, baseline) and simple trigonometry (triangulation) to calculate the 3D position (including depth) of the point in the real world.
Prerequisites: Gearing Up for 3D Vision
Let's gather the tools and software needed for this project.
Required Hardware:
NVIDIA Jetson Board: A Jetson Nano Developer Kit (good for starting) or a more powerful Jetson AGX Xavier/Orin (for demanding applications).
Stereo Camera Setup:
Option 1 (Integrated): An IMX219-based stereo camera module (like those from Waveshare or Arducam) designed for Jetson. These often come pre-mounted with a fixed baseline.
Option 2 (DIY): Two individual CSI cameras (e.g., Raspberry Pi Camera Module V2, which uses the IMX219 sensor) compatible with your Jetson. You'll need to create a rigid mount to keep them at a fixed distance and orientation. Ensure you have the correct ribbon cables for your Jetson's CSI ports.
Power Supply: Adequate power supply for your Jetson board (check the specs!).
MicroSD Card: A high-quality card (32GB or larger, Class 10/U1/A1 minimum) flashed with JetPack.
(Optional but Recommended) USB Keyboard, Mouse, Monitor for setup.
(Optional) A 3D printed or sturdy mount for your cameras if using individual ones.
(Optional) A checkerboard pattern printed out for calibration.
Required Software:
NVIDIA JetPack SDK: The operating system and core libraries for Jetson. Install the latest version compatible with your board. Includes Linux for Tegra (L4T), CUDA, cuDNN, TensorRT, and OpenCV pre-optimized for Jetson.
Python 3: Usually included with JetPack.
OpenCV: Version 4.5 or later (often included in JetPack, but verify). Crucial for image processing and stereo vision algorithms.
NumPy: For numerical operations.
jetmulticam Library: Simplifies capturing streams from multiple CSI cameras on Jetson.
(Optional) Open3D: For advanced 3D point cloud visualization and processing.
(Optional) TensorFlow/PyTorch: If you plan to integrate deep learning models later.
Setup Instructions:
Flash JetPack: Follow the official NVIDIA instructions to flash the JetPack SDK onto your microSD card and boot up your Jetson: NVIDIA JetPack SDK. Complete the initial OS setup.
Connect Peripherals: Attach keyboard, mouse, monitor (or set up headless access via SSH).
Install System Dependencies & OpenCV (if needed): JetPack usually includes OpenCV, but let's ensure essentials are present and update the package list.
sudo apt update sudo apt install -y python3-pip python3-dev build-essential cmake git # Verify OpenCV installation (usually pre-installed with JetPack) python3 -c "import cv2; print(f'OpenCV Version: {cv2.__version__}')" # If OpenCV is missing or the wrong version, you might need to build it from source (a lengthy process!) # or find a suitable pre-built package for your JetPack version.
Install jetmulticam: This library makes multi-camera handling much easier.
# Install build dependency sudo apt install -y python3-cython # Clone the repository git clone https://github.com/NVIDIA-AI-IOT/jetson-multicamera-pipelines.git cd jetson-multicamera-pipelines # Install library dependencies sudo bash scripts/install_dependencies.sh # Build and install the library sudo pip3 install Cython numpy # Ensure Cython and numpy are installed via pip too sudo python3 setup.py build_ext --inplace sudo python3 setup.py install cd .. # Go back to your main project directory
Note: Installation steps for libraries can sometimes change. Refer to the official jetmulticam repository if you encounter issues.
(Optional) Install Open3D:
pip3 install open3d # Note: Pre-built Open3D wheels for Jetson/ARM64 might not always be available. # You might need to build it from source, which can be complex. Check Open3D docs.
Implementation Guide: Building Your Stereo Vision Pipeline
Let's build the system step-by-step.
Step 1: Setting Up the Hardware
Power Down: Ensure your Jetson is completely powered off.
Connect Cameras: Carefully connect your stereo camera module or individual cameras to the CSI ports on the Jetson board. Pay close attention to the ribbon cable orientation – usually, the blue tab faces away from the PCB or towards the Ethernet port side, but check your specific board and camera documentation. Ensure the connectors are securely latched.
If using individual cameras, mount them rigidly with a known baseline (e.g., 6-12 cm apart). They should be parallel and aligned as closely as possible.
Power Up: Connect the power supply and boot the Jetson.
Verify Camera Detection: Open a terminal and test if the system detects the cameras. A common way is using gst-launch-1.0 or a simple OpenCV script. For jetmulticam, it often assumes cameras are at /dev/video0, /dev/video1, etc. You can list devices:
ls /dev/video*
You should see entries corresponding to your connected cameras.
Step 2: The Crucial Step - Camera Calibration
Why Calibrate? Every camera lens has imperfections (distortions), and we need to know the exact geometric relationship between the two cameras (rotation and translation) and their internal parameters (focal length, principal point). Without calibration, your depth measurements will be inaccurate.
How to Calibrate: The standard method uses a checkerboard pattern.
Get a Checkerboard: Print a checkerboard pattern (e.g., 9x6 squares) on a flat, rigid surface. Measure the size of one square accurately (e.g., 25mm).
Capture Calibration Images: Write a script to capture simultaneous image pairs from both cameras. Show the checkerboard to the cameras from various angles, distances, and positions, ensuring it fills a good portion of the frame in many shots. Capture maybe 20-30 good pairs.
# Example snippet for capturing calibration pairs import cv2 from jetmulticam import CameraPipeline import time import os # --- Calibration Parameters --- CHECKERBOARD = (6, 9) # Inner corners count (height, width) SAVE_DIR = "calibration_images" FRAME_WIDTH = 640 # Adjust as needed FRAME_HEIGHT = 480 # Adjust as needed # --- --- if not os.path.exists(SAVE_DIR): os.makedirs(SAVE_DIR) os.makedirs(os.path.join(SAVE_DIR, "left")) os.makedirs(os.path.join(SAVE_DIR, "right")) # Initialize cameras (assuming IDs 0 and 1) # Adjust width/height/framerate as supported by your cameras pipeline = CameraPipeline( [0, 1], capture_width=FRAME_WIDTH, capture_height=FRAME_HEIGHT, display_width=FRAME_WIDTH, display_height=FRAME_HEIGHT, framerate=30, use_display=False # We just want to save frames ) img_count = 0 print("Press 'c' to capture image pair, 'q' to quit.") # Create windows for preview (optional but helpful) cv2.namedWindow("Left Camera", cv2.WINDOW_NORMAL) cv2.namedWindow("Right Camera", cv2.WINDOW_NORMAL) while True: img_left = pipeline.read(0) img_right = pipeline.read(1) if img_left is None or img_right is None: print("Error reading frame, skipping.") time.sleep(0.1) continue cv2.imshow("Left Camera", img_left) cv2.imshow("Right Camera", img_right) key = cv2.waitKey(1) & 0xFF if key == ord('c'): # Find checkerboard corners (optional preview) gray_left = cv2.cvtColor(img_left, cv2.COLOR_BGR2GRAY) gray_right = cv2.cvtColor(img_right, cv2.COLOR_BGR2GRAY) ret_l, corners_l = cv2.findChessboardCorners(gray_left, CHECKERBOARD, None) ret_r, corners_r = cv2.findChessboardCorners(gray_right, CHECKERBOARD, None) if ret_l and ret_r: left_name = os.path.join(SAVE_DIR, "left", f"left_{img_count:02d}.png") right_name = os.path.join(SAVE_DIR, "right", f"right_{img_count:02d}.png") cv2.imwrite(left_name, img_left) cv2.imwrite(right_name, img_right) print(f"Captured pair {img_count}") img_count += 1 else: print("Checkerboard not found in both images. Try again.") elif key == ord('q'): break pipeline.release() cv2.destroyAllWindows() print(f"Captured {img_count} image pairs.")
Run Stereo Calibration: Use OpenCV's stereoCalibrate function. This is computationally intensive.
# Example snippet for running calibration (run after capturing images) import cv2 import numpy as np import glob import os # --- Calibration Parameters --- CHECKERBOARD = (6, 9) # Inner corners count (height, width) SQUARE_SIZE_MM = 25 # Size of one checkerboard square in mm IMAGE_DIR = "calibration_images" FRAME_WIDTH = 640 # Must match captured image size FRAME_HEIGHT = 480 # Must match captured image size CALIBRATION_FILE = "stereo_calibration.npz" # --- --- # Termination criteria for corner refinement criteria = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 30, 0.001) # Prepare object points (0,0,0), (1,0,0), (2,0,0) ....,(6,5,0) objp = np.zeros((CHECKERBOARD[0] * CHECKERBOARD[1], 3), np.float32) objp[:, :2] = np.mgrid[0:CHECKERBOARD[1], 0:CHECKERBOARD[0]].T.reshape(-1, 2) objp = objp * SQUARE_SIZE_MM # Scale to real-world size # Arrays to store object points and image points from all images. objpoints = [] # 3d point in real world space imgpoints_left = [] # 2d points in left image plane. imgpoints_right = [] # 2d points in right image plane. images_left = sorted(glob.glob(os.path.join(IMAGE_DIR, 'left', '*.png'))) images_right = sorted(glob.glob(os.path.join(IMAGE_DIR, 'right', '*.png'))) if not images_left or len(images_left) != len(images_right): print("Error: Mismatched or missing calibration images.") exit() print(f"Found {len(images_left)} image pairs. Processing...") for i, (fname_left, fname_right) in enumerate(zip(images_left, images_right)): img_l = cv2.imread(fname_left) img_r = cv2.imread(fname_right) gray_l = cv2.cvtColor(img_l, cv2.COLOR_BGR2GRAY) gray_r = cv2.cvtColor(img_r, cv2.COLOR_BGR2GRAY) # Find the chess board corners ret_l, corners_l = cv2.findChessboardCorners(gray_l, CHECKERBOARD, None) ret_r, corners_r = cv2.findChessboardCorners(gray_r, CHECKERBOARD, None) # If found, add object points, image points (after refining them) if ret_l and ret_r: print(f"Processing pair {i+1}...") objpoints.append(objp) corners2_l = cv2.cornerSubPix(gray_l, corners_l, (11, 11), (-1, -1), criteria) imgpoints_left.append(corners2_l) corners2_r = cv2.cornerSubPix(gray_r, corners_r, (11, 11), (-1, -1), criteria) imgpoints_right.append(corners2_r) # Draw and display the corners (optional visualization) # cv2.drawChessboardCorners(img_l, CHECKERBOARD, corners2_l, ret_l) # cv2.drawChessboardCorners(img_r, CHECKERBOARD, corners2_r, ret_r) # cv2.imshow(f'Corners {i}', np.hstack((img_l, img_r))) # cv2.waitKey(50) else: print(f"Warning: Checkerboard not found in pair {i+1}. Skipping.") cv2.destroyAllWindows() if not objpoints: print("Calibration failed. No valid checkerboard pairs found.") exit() print("\nPerforming single camera calibrations...") # Calibrate each camera individually first ret_l, mtx_l, dist_l, rvecs_l, tvecs_l = cv2.calibrateCamera(objpoints, imgpoints_left, gray_l.shape[::-1], None, None) print("Left camera calibrated.") ret_r, mtx_r, dist_r, rvecs_r, tvecs_r = cv2.calibrateCamera(objpoints, imgpoints_right, gray_r.shape[::-1], None, None) print("Right camera calibrated.") print("\nPerforming stereo calibration...") # Stereo calibration flags = cv2.CALIB_FIX_INTRINSIC # Fix intrinsic parameters obtained from single calibration # Or try flags = 0 for joint optimization criteria_stereo = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 100, 1e-5) ret_stereo, M1, d1, M2, d2, R, T, E, F = cv2.stereoCalibrate( objpoints, imgpoints_left, imgpoints_right, mtx_l, dist_l, mtx_r, dist_r, gray_l.shape[::-1], # Use shape of one of the gray images criteria=criteria_stereo, flags=flags ) if ret_stereo: print("\nStereo calibration successful!") print("RMS Error:", ret_stereo) print("Saving calibration results to", CALIBRATION_FILE) # Stereo rectification (computes rectification transforms) R1, R2, P1, P2, Q, roi_left, roi_right = cv2.stereoRectify( M1, d1, M2, d2, gray_l.shape[::-1], R, T, flags=cv2.CALIB_ZERO_DISPARITY, alpha=0.9 # alpha=0 crops tightly, alpha=1 keeps all pixels ) # Save all parameters np.savez(CALIBRATION_FILE, mtx_left=M1, dist_left=d1, R1=R1, P1=P1, mtx_right=M2, dist_right=d2, R2=R2, P2=P2, Q=Q, T=T, R=R, # Also save R & T if needed roi_left=roi_left, roi_right=roi_right) print("Calibration data saved.") else: print("Stereo calibration failed.") # You can also print the matrices: # print("\nLeft Camera Matrix (M1):\n", M1) # print("\nLeft Distortion Coefficients (d1):\n", d1) # ... and so on for M2, d2, R, T, Q etc.
Save Calibration Data: The script above saves the crucial matrices (M1, d1, M2, d2, R, T, R1, R2, P1, P2, Q) to a .npz file. We'll load this file in our main application. Guard this file! Re-calibration is only needed if the cameras move relative to each other.
Step 3: Capturing Synchronized Video Streams
Now we use jetmulticam to grab frames from both cameras efficiently.
# Part of your main application script
import cv2
from jetmulticam import CameraPipeline
import numpy as np
import time # To measure FPS
# Load calibration data
CALIBRATION_FILE = "stereo_calibration.npz"
try:
calib_data = np.load(CALIBRATION_FILE)
mtx_left = calib_data['mtx_left']
dist_left = calib_data['dist_left']
R1 = calib_data['R1']
P1 = calib_data['P1']
mtx_right = calib_data['mtx_right']
dist_right = calib_data['dist_right']
R2 = calib_data['R2']
P2 = calib_data['P2']
Q = calib_data['Q']
roi_left = calib_data['roi_left'] # Region of interest after rectification
roi_right = calib_data['roi_right']
print("Calibration data loaded successfully.")
except FileNotFoundError:
print(f"Error: Calibration file '{CALIBRATION_FILE}' not found.")
print("Please run the calibration script first.")
exit()
except Exception as e:
print(f"Error loading calibration file: {e}")
exit()
# --- Camera/Pipeline Parameters ---
CAM_IDS = [0, 1] # Check /dev/video* if these are correct
FRAME_WIDTH = 640 # Should match calibration image size
FRAME_HEIGHT = 480 # Should match calibration image size
FRAMERATE = 30 # Adjust based on camera capability & desired speed
# --- ---
print("Initializing camera pipeline...")
pipeline = CameraPipeline(
CAM_IDS,
capture_width=FRAME_WIDTH,
capture_height=FRAME_HEIGHT,
display_width=FRAME_WIDTH, # Output size can differ if needed
display_height=FRAME_HEIGHT,
framerate=FRAMERATE,
use_display=False # Set True if you want jetmulticam's internal display
)
print("Pipeline initialized.")
# Pre-compute rectification maps (do this once)
map1_left, map2_left = cv2.initUndistortRectifyMap(mtx_left, dist_left, R1, P1, (FRAME_WIDTH, FRAME_HEIGHT), cv2.CV_16SC2)
map1_right, map2_right = cv2.initUndistortRectifyMap(mtx_right, dist_right, R2, P2, (FRAME_WIDTH, FRAME_HEIGHT), cv2.CV_16SC2)
print("Rectification maps computed.")
# --- Main Loop ---
while True:
start_time = time.time() # Start timer for FPS calculation
img_left_raw = pipeline.read(CAM_IDS[0])
img_right_raw = pipeline.read(CAM_IDS[1])
if img_left_raw is None or img_right_raw is None:
print("Error: Failed to capture frame(s).")
time.sleep(0.1) # Avoid busy-waiting
if not pipeline.running: # Check if pipeline stopped
break
continue
# --- Rectify Images ---
img_left_rect = cv2.remap(img_left_raw, map1_left, map2_left, cv2.INTER_LINEAR)
img_right_rect = cv2.remap(img_right_raw, map1_right, map2_right, cv2.INTER_LINEAR)
# --- (Proceed to Step 4: Depth Calculation) ---
# ... Depth calculation code will go here ...
# --- Display Rectified Images (Optional) ---
# Crop to region of interest if needed (removes black borders)
# x, y, w, h = roi_left
# img_left_rect_cropped = img_left_rect[y:y+h, x:x+w]
# x, y, w, h = roi_right
# img_right_rect_cropped = img_right_rect[y:y+h, x:x+w]
cv2.imshow("Rectified Left", img_left_rect)
cv2.imshow("Rectified Right", img_right_rect)
# --- Calculate and Display FPS ---
end_time = time.time()
fps = 1.0 / (end_time - start_time)
print(f"FPS: {fps:.2f}") # Print FPS to console
key = cv2.waitKey(1) & 0xFF
if key == ord('q'):
break
# --- End Main Loop ---
print("Releasing pipeline...")
pipeline.release()
cv2.destroyAllWindows()
print("Done.")
Step 4: Implementing Depth Calculation
Now for the core logic: calculating disparity and converting it to depth.
Convert to Grayscale: Disparity algorithms typically work on single-channel images.
Compute Disparity: We'll use OpenCV's StereoBM (Block Matching) algorithm for simplicity and speed, though StereoSGBM (Semi-Global Block Matching) often provides better accuracy at a higher computational cost.
Filter Disparity (Optional but Recommended): Raw disparity maps are often noisy. Filters like WLS (Weighted Least Squares) can significantly improve quality.
Reproject to 3D: Use the Q matrix (from stereoRectify) with reprojectImageTo3D to convert the disparity map into a 3D point cloud. The Q matrix encodes the geometry needed for this projection.
# --- Add inside the main loop, after rectification ---
# --- Convert to Grayscale ---
gray_left = cv2.cvtColor(img_left_rect, cv2.COLOR_BGR2GRAY)
gray_right = cv2.cvtColor(img_right_rect, cv2.COLOR_BGR2GRAY)
# --- Compute Disparity ---
# StereoBM parameters need tuning based on your setup and environment
# numDisparities: Must be divisible by 16. Larger values find larger disparities (closer objects).
# blockSize: Must be odd. Size of the matching block. Larger values smooth disparity but lose detail.
stereo = cv2.StereoBM_create(numDisparities=64, blockSize=15)
# You can also try StereoSGBM for better results (more computationally expensive)
# stereo = cv2.StereoSGBM_create(minDisparity=0, numDisparities=64, blockSize=5, ...) # Many params to tune!
disparity_raw = stereo.compute(gray_left, gray_right).astype(np.float32) / 16.0 # Divide by 16
# --- Post-processing/Filtering Disparity (Example using simple normalization for display) ---
# For better results, consider using cv2.ximgproc.createDisparityWLSFilter
# Normalize disparity map for visualization (optional)
disparity_display = cv2.normalize(disparity_raw, None, alpha=0, beta=255, norm_type=cv2.NORM_MINMAX, dtype=cv2.CV_8U)
# --- Calculate Depth Map (3D Point Cloud) ---
# Note: disparity_raw contains disparities, not depths yet
# Avoid dividing by zero or invalid disparities
mask_valid = disparity_raw > stereo.getMinDisparity() # Use the actual minDisparity if not 0
points_3D = cv2.reprojectImageTo3D(disparity_raw, Q, handleMissingValues=True)
# Set invalid points (where disparity was 0 or invalid) to Nan or a specific value
points_3D[~mask_valid] = [np.nan, np.nan, np.nan]
# Extract depth (Z coordinate) - The units depend on your SQUARE_SIZE_MM in calibration
# If SQUARE_SIZE_MM was in mm, depth will be in mm.
depth_map = points_3D[:, :, 2]
# --- Display Disparity Map ---
cv2.imshow("Disparity Map", disparity_display)
# You can now use 'depth_map' or 'points_3D' for further processing
# For example, get depth at the center pixel:
# center_x, center_y = FRAME_WIDTH // 2, FRAME_HEIGHT // 2
# depth_at_center = depth_map[center_y, center_x]
# if not np.isnan(depth_at_center) and not np.isinf(depth_at_center):
# print(f"Depth at center: {depth_at_center:.2f} mm") # Assuming mm units
# --- (Rest of the loop: display FPS, waitKey, etc.) ---
# ...
Common Challenges and Solutions
Camera Synchronization:
Problem: Frames captured at slightly different times cause incorrect disparity.
Solution: jetmulticam generally handles this well by using GStreamer's synchronization mechanisms. Hardware synchronization (triggering cameras simultaneously) offers the best results but requires specific hardware. Check timestamps if implementing custom capture.
Lighting Conditions:
Problem: Poor lighting, shadows, glare, or uniform/textureless surfaces make it hard for the algorithm to find matching points.
Solution: Ensure good, consistent lighting. Avoid direct sunlight or reflections if possible. Active illumination (e.g., an infrared projector casting a pattern) can help with textureless surfaces (like Kinect). Adjust camera exposure settings.
Calibration Accuracy:
Problem: Inaccurate calibration leads to warped disparity/depth maps and incorrect measurements.
Solution: Use a high-quality, flat checkerboard. Capture images from many diverse viewpoints. Ensure cornerSubPix refines corners accurately. Check the RMS error reported by stereoCalibrate (lower is better, < 0.5 pixels is usually good). Recalibrate if the cameras are bumped or moved.
Performance Bottlenecks:
Problem: Stereo processing (especially SGBM or filtering) can be slow, limiting frame rate.
Solution: Use StereoBM for speed. Tune parameters (numDisparities, blockSize). Reduce image resolution (recalibrate if you do!). Leverage Jetson's GPU (see Advanced Techniques). Profile your code to find bottlenecks.
Disparity Range:
Problem: Objects too close or too far might be outside the calculated disparity range (numDisparities).
Solution: Adjust numDisparities based on your expected working distance and baseline. A wider baseline helps with far objects but can lose near objects; a narrower baseline is better for near objects.
Advanced Techniques & Optimization
Pushing the performance envelope on the Jetson:
GPU Acceleration with OpenCV: OpenCV built with CUDA support (usually default in JetPack) has GPU implementations for some functions. Check cv2.cuda module. For example, cv2.cuda.StereoBM or cv2.cuda.remap. This requires transferring data to/from the GPU (cv2.cuda_GpuMat).
TensorRT for AI Models: If integrating object detection or segmentation, convert your models (TensorFlow, PyTorch) to TensorRT for significant inference speedup on the Jetson GPU/DLA.
Algorithm Choice: StereoBM is fast but basic. StereoSGBM is better but slower. Explore other methods like ELAS or learning-based stereo methods if performance allows.
Parameter Tuning: Carefully tune StereoBM/SGBM parameters (numDisparities, blockSize, uniquenessRatio, speckleWindowSize, speckleRange). This is often trial-and-error based on your scene.
Resolution vs. Speed Trade-off: Lowering the camera resolution significantly speeds up processing but reduces detail and potentially accuracy. Find the sweet spot for your application. Remember to recalibrate if you change resolution.
Threading/Multiprocessing: Perform capture, rectification, disparity calculation, and post-processing in separate threads or processes to utilize multiple CPU cores or run tasks in parallel with GPU operations. Python's threadingor multiprocessing modules can be used.
Benchmarking: Measuring Performance
How well does our system perform? Let's measure it.
Methodology:
Frame Rate (FPS): Measure the time taken for one full iteration of the main loop (capture -> rectify -> disparity -> depth -> display). FPS = 1 / iteration_time. Average over many frames. We added a basic FPS calculation in the sample code.
CPU/GPU Utilization: Use the tegrastats utility in the Jetson terminal while your script is running. It shows CPU core usage, GPU utilization, memory usage, etc.
sudo tegrastats
Depth Accuracy: This is harder. Requires ground truth depth data (e.g., from a Lidar sensor or known object distances). Calculate metrics like Root Mean Square Error (RMSE) or Mean Absolute Error (MAE) between your calculated depth and the ground truth. Qualitatively, observe if distances look reasonable for objects at known positions.
Example Results (Illustrative):
Metric | Basic StereoBM(CPU) | Optimized (StereoBM, Lower Res, Tuned) | Potential w/ GPU StereoBM/SGBM |
Frame Rate (FPS) | ~10-15 FPS @ 640x480 | ~25-30 FPS @ 320x240 | Potentially 30+ FPS |
Depth Quality | Noisy, basic | Smoother, acceptable | Can be significantly better |
CPU Utilization (%) | High (e.g., 70-90%) | Moderate (e.g., 40-60%) | Lower CPU (GPU takes load) |
GPU Utilization (%) | Low | Low | Moderate to High |
Note: These are rough estimates. Actual performance depends heavily on Jetson model, camera resolution, specific parameters, and scene complexity.
Interpretation:
Benchmarking helps identify bottlenecks. High CPU usage with basic StereoBM suggests CPU is the limit. Optimization techniques like reducing resolution or moving to GPU implementations can shift the load and improve FPS. There's always a trade-off between speed, accuracy, and resolution.
Industry Applications: Where Stereo Vision Shines
The ability to perceive depth opens doors:
Autonomous Vehicles: Cars like those from Waymo and others use stereo cameras (often alongside Lidar and Radar) for object detection, distance estimation, lane keeping, and creating 3D maps of the surroundings.
Robotics: Warehouse robots use stereo vision for navigating aisles, identifying shelves, and picking objects accurately. Mobile robots use it for obstacle avoidance and SLAM (Simultaneous Localization and Mapping).
Drones: Autonomous drones rely on stereo vision for safe navigation in complex environments (like forests or indoors), obstacle avoidance, terrain mapping, and precision landing.
Augmented Reality (AR): Stereo vision helps map the real world, allowing virtual objects to be placed realistically and interact correctly with real surfaces.
Medical Imaging: Endoscopic stereo cameras provide surgeons with depth perception inside the human body, improving precision.
Industrial Inspection: Measuring object dimensions, checking assembly correctness, and guiding robotic arms in manufacturing processes.
Conclusion: Your Journey into 3D Vision
You've now walked through the entire process of building a functional stereo vision system on an NVIDIA Jetson! From connecting cameras and calibrating them meticulously to capturing frames, calculating disparity, and generating depth maps, you have the foundational knowledge to "hack" together 3D perception for your edge AI projects.
Stereo vision is a rich field with ongoing research. Future trends include deeper integration with AI for semantic understanding of the 3D scene, improvements in real-time performance through dedicated hardware/algorithms, and the use of event-based cameras for high-speed scenarios.
Experiment with different parameters, try the StereoSGBM algorithm, explore filtering techniques, visualize the 3D point cloud with Open3D, and perhaps combine depth data with object detection. The world – in 3D – is yours to explore!
References & Further Reading
OpenCV Documentation:
Camera Calibration: docs.opencv.org/4.x/d9/d0c/group__calib3d.html
Stereo Matching: docs.opencv.org/4.x/dd/d53/tutorial_py_depthmap.html
jetson-multicamera-pipelines Repository: github.com/NVIDIA-AI-IOT/jetson-multicamera-pipelines
NVIDIA Jetson Developer Forums: A great place for specific questions and community support: forums.developer.nvidia.com/c/agx-autonomous-machines/jetson-embedded-systems/70
Related Articles
Article Info
Engage
Table of Contents
- Introduction: Seeing the World in 3D with Jetson
- Understanding the Magic: Core Stereo Vision Concepts
- Prerequisites: Gearing Up for 3D Vision
- Implementation Guide: Building Your Stereo Vision Pipeline
- Step 1: Setting Up the Hardware
- Step 2: The Crucial Step - Camera Calibration
- Step 3: Capturing Synchronized Video Streams
- Step 4: Implementing Depth Calculation
- Common Challenges and Solutions
- Advanced Techniques & Optimization
- Benchmarking: Measuring Performance
- Industry Applications: Where Stereo Vision Shines
- Conclusion: Your Journey into 3D Vision
- References & Further Reading